统计字符串中出现最多的字母java
时间: 2023-08-10 16:02:20 浏览: 88
可以使用HashMap来统计字符串中出现最多的字母。
具体实现流程如下:
1. 创建一个HashMap用于存储每个字母出现的次数。
2. 遍历字符串中的每个字符,如果该字符在HashMap中不存在,则将该字符作为key,值为1存入HashMap中;否则将该字符对应的value加1。
3. 遍历完字符串后,遍历HashMap,找出出现次数最多的字符。
4. 返回出现次数最多的字符。
下面是Java代码实现:
```java
public static char getMaxOccurringChar(String str) {
if (str == null || str.isEmpty()) {
throw new IllegalArgumentException("Input cannot be null or empty");
}
Map<Character, Integer> charCountMap = new HashMap<>();
char[] charArray = str.toCharArray();
for (char ch : charArray) {
if (charCountMap.containsKey(ch)) {
charCountMap.put(ch, charCountMap.get(ch) + 1);
} else {
charCountMap.put(ch, 1);
}
}
char maxChar = ' ';
int maxCount = 0;
for (Map.Entry<Character, Integer> entry : charCountMap.entrySet()) {
if (entry.getValue() > maxCount) {
maxChar = entry.getKey();
maxCount = entry.getValue();
}
}
return maxChar;
}
```
调用示例:
```java
String str = "hello world";
char maxChar = getMaxOccurringChar(str);
System.out.println("出现最多的字符是:" + maxChar);
```
阅读全文
相关推荐
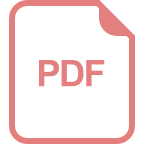















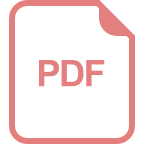