爬取腾讯新闻网新闻(https://new.qq.com/)及清洗 爬取要闻、北京、财经、科技、娱乐、国际、军事、游戏、乐活模块新闻数据,每个模块爬取100条带图片的数据;爬取的字段包括:url、标题、发布时间、新闻来源、发布地点、新闻内容;数据清洗的要求:发布时间清洗为标准日期格式(yyyy-mm-dd HH:MM:SS),发布地点只保留地点信息(不要“发布于xxx”)。文本信息保存为csv格式(带表头)。
时间: 2023-07-10 10:05:21 浏览: 66
好的,这个任务可以使用Python中的requests和BeautifulSoup模块来完成。以下是实现代码,需要安装相应的模块:
```python
import requests
from bs4 import BeautifulSoup
import re
import csv
# 定义一个函数,用于对发布时间进行格式化
def format_time(time_str):
# 时间字符串的正则表达式
pattern = re.compile(r'\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}')
# 使用正则表达式匹配时间字符串
match = pattern.search(time_str)
if match:
# 如果匹配成功,返回标准格式的时间字符串
return match.group()
else:
# 否则返回空字符串
return ''
# 定义一个函数,用于爬取一个模块的新闻数据
def crawl_news(url, module):
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 解析HTML页面
soup = BeautifulSoup(response.content, 'html.parser')
# 获取新闻列表
news_list = soup.find_all('div', {'class': 'detail'})
# 定义一个列表,用于保存新闻数据
data = []
# 遍历新闻列表,提取所需的字段
for news in news_list:
# 获取新闻标题
title = news.find('a').text.strip()
# 获取新闻链接
news_url = news.find('a')['href']
# 获取新闻发布时间
time_str = news.find('span', {'class': 'time'}).text
publish_time = format_time(time_str)
# 获取新闻来源和发布地点
source_str = news.find('span', {'class': 'source'}).text
source_list = source_str.split(' ')
if len(source_list) > 1:
source = source_list[0]
location = source_list[1].replace('发布于', '').strip()
else:
source = source_list[0]
location = ''
# 获取新闻内容
content = ''
content_response = requests.get(news_url)
content_soup = BeautifulSoup(content_response.content, 'html.parser')
paragraphs = content_soup.find_all('p', {'class': 'text'})
for p in paragraphs:
content += p.text.strip() + '\n'
# 获取新闻图片链接
image_url = ''
image = news.find('img')
if image:
image_url = image['src']
# 将提取的字段保存到列表中
data.append([news_url, title, publish_time, source, location, content, image_url])
# 将数据保存到CSV文件中
with open(f'{module}.csv', 'w', newline='', encoding='utf-8-sig') as f:
writer = csv.writer(f)
writer.writerow(['url', 'title', 'publish_time', 'source', 'location', 'content', 'image'])
writer.writerows(data)
# 定义一个函数,用于爬取所有模块的新闻数据
def crawl_all_news():
# 定义模块和对应的URL
modules = {
'要闻': 'https://new.qq.com/ch/top/',
'北京': 'https://new.qq.com/ch/BJ/',
'财经': 'https://new.qq.com/ch/finance/',
'科技': 'https://new.qq.com/ch/tech/',
'娱乐': 'https://new.qq.com/ch/ent/',
'国际': 'https://new.qq.com/ch/world/',
'军事': 'https://new.qq.com/ch/milite/',
'游戏': 'https://new.qq.com/ch/games/',
'乐活': 'https://new.qq.com/ch/life/'
}
# 遍历所有模块,调用crawl_news函数爬取数据
for module, url in modules.items():
crawl_news(url, module)
```
使用`crawl_all_news`函数即可爬取所有模块的新闻数据,数据保存在对应的CSV文件中。
相关推荐
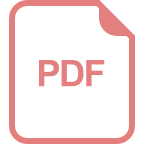
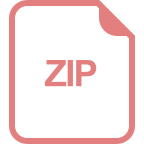
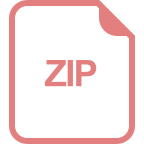














