vector<PointVectorPair> points; for (size_t i = 0; i < input->size(); i++) { float px = input->points[i].x; float py = input->points[i].y; float pz = input->points[i].z; float nx = input->points[i].normal_x; float ny = input->points[i].normal_y; float nz = input->points[i].normal_z; points.push_back(PointVectorPair(Kernel::Point_3(px, py, pz), Kernel::Vector_3(nx, ny, nz))); } // ---------------------------------参数设置--------------------------------- const double s_angle = 25; // 平滑度,值越大越平滑,取值范围[0,90] const double edge_s = 0; // 边缘的敏感性取值范围[0,1] const double n_radius = 0.25; // 邻域点搜索半径 const std::size_t n_out = points.size() * 10; // 上采样后的点数 // ----------------------------------上采样---------------------------------- CGAL::edge_aware_upsample_point_set<CGAL::Parallel_if_available_tag>(points, std::back_inserter(points), CGAL::parameters::point_map(CGAL::First_of_pair_property_map<PointVectorPair>()). normal_map(CGAL::Second_of_pair_property_map<PointVectorPair>()). sharpness_angle(s_angle). // 控制输出结果的平滑度,值越大越平滑,取值范围[0,90]。 edge_sensitivity(edge_s). // 边缘的敏感性取值范围[0,1],边缘敏感度越大,在尖锐边缘处插入点的有限度越高 neighbor_radius(n_radius). // 邻域点的个数,如果提供,查询点的邻域是用一个固定的球面计算的半径 // 而不是固定数量的邻居。在这种情况下,参数k用于限制每个球邻域返回点的数量(避免高密度区域的点过多) number_of_output_points(n_out)); // 上采样后的点数 std::cout << "上采样完毕!!!" << std::endl;将上述代码中的CGAL带有法线的点云数据转换PCL库点云数据PointNormal
时间: 2023-08-15 08:07:15 浏览: 323
上述代码片段中,使用了CGAL库的`edge_aware_upsample_point_set`函数对带有法线的点云数据进行上采样,并将结果存储在`points`向量中。现在你想将这些点转换为PCL库中的PointNormal类型的点云数据。
你可以按照以下步骤修改代码,将`points`向量中的点转换为PCL库中的PointNormal类型的点云数据:
```cpp
#include <pcl/point_types.h>
#include <pcl/point_cloud.h>
// 创建PCL库中的PointNormal类型的点云数据
pcl::PointCloud<pcl::PointNormal>::Ptr pcl_cloud(new pcl::PointCloud<pcl::PointNormal>);
for (const auto& point : points)
{
pcl::PointNormal pcl_point;
pcl_point.x = point.first.x();
pcl_point.y = point.first.y();
pcl_point.z = point.first.z();
pcl_point.normal_x = point.second.x();
pcl_point.normal_y = point.second.y();
pcl_point.normal_z = point.second.z();
pcl_cloud->push_back(pcl_point);
}
// 保存PCL点云数据
pcl::io::savePLYFile("output.ply", *pcl_cloud);
```
在上述代码中,我们根据`points`向量中的每个点的位置和法线信息,创建了相应的PCL库中的PointNormal类型的点云数据。最后,我们将转换后的点云数据保存为"output.ply"文件。
请确保已正确安装和配置PCL库,并将相关头文件和库文件包含到你的项目中。这样,你就可以使用上述代码将CGAL中的点云数据转换为PCL库中的PointNormal类型的点云数据了。
阅读全文
相关推荐
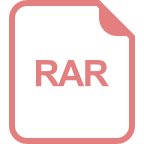

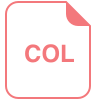
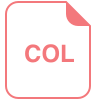
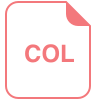
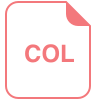
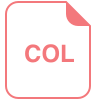






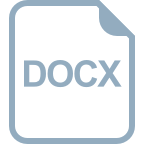
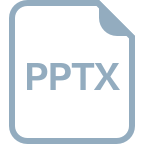
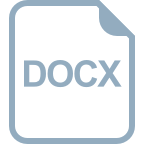