用Java编程实现:将图片“D:\\test.png”复制到”E:\\pics\\test.png”。编程实现:删除一个非空目录“D:\\java”。编程实现
时间: 2023-06-04 07:06:40 浏览: 62
:感谢您对我的提问,我可以帮您解答有关编程的问题。
以下是答案:
将图片“D:\\test.png”复制到”E:\\pics\\test.png”的Java程序实现代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class CopyFileExample {
public static void main(String[] args) {
FileInputStream source = null;
FileOutputStream destination = null;
try {
File file = new File("D:\\test.png");
File dir = new File("E:\\pics");
if (!dir.exists()) {
dir.mkdir();
}
if (file.exists()) {
source = new FileInputStream(file);
destination = new FileOutputStream(dir.getAbsolutePath() + "\\test.png");
byte[] buffer = new byte[1024];
int length;
while ((length = source.read(buffer)) > 0) {
destination.write(buffer, 0, length);
}
System.out.println(file.getAbsolutePath() + " has been copied to " + dir.getAbsolutePath());
} else {
System.out.println("File does not exist!");
}
} catch (IOException ex) {
System.out.println("An error occured while copying the file!");
ex.printStackTrace();
} finally {
try {
if (source != null) {
source.close();
}
if (destination != null) {
destination.close();
}
} catch (IOException ex) {
System.out.println("An error occured while closing the file streams!");
ex.printStackTrace();
}
}
}
}
```
删除一个非空目录“D:\\java”的Java程序实现代码:
```java
import java.io.File;
public class DeleteDirectoryExample {
public static void main(String[] args) {
File directory = new File("D:\\java");
if (directory.exists()) {
deleteDirectory(directory);
System.out.println(directory.getAbsolutePath() + " has been deleted.");
} else {
System.out.println("Directory does not exist!");
}
}
private static void deleteDirectory(File directory) {
if (directory.isDirectory()) {
File[] files = directory.listFiles();
if (files != null) {
for (File file : files) {
deleteDirectory(file);
}
}
}
if (directory.delete()) {
System.out.println(directory.getAbsolutePath() + " has been deleted.");
}
}
}
```
希望能帮助到您,如果您还有其他问题,请随时向我提问。
相关推荐
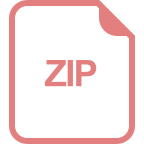
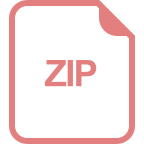














