语句goods.sort(key=lambda obj: obj.value / obj.weight, reverse=True) for i in goods: if sum_weight + i.weight <= W : sum_weight = sum_weight + i.weight ppp = ppp + i.value danjia = danjia + i.value / i.weight result.append(i.weight) return result, sum_weight, ppp, danjia分别是什么意思
时间: 2024-04-27 16:24:13 浏览: 96
这段代码是贪心算法的核心部分。其中`goods.sort(key=lambda obj: obj.value / obj.weight, reverse=True)`是按照单位重量价值从大到小对物品列表进行排序。`for i in goods:`是对物品列表进行遍历,然后`if sum_weight + i.weight <= W :`表示如果当前物品可以放入背包,则将其放入背包,同时更新背包的总重量`sum_weight`、总价值`ppp`和平均价值`danjia`,并将物品的重量`i.weight`加入选中物品的重量列表`result`中。最后,函数返回选中物品的重量列表、总重量、总价值和平均价值,这些信息可以用于衡量该贪心算法的效果。
相关问题
class Greedy(object): def greedy(self, goods, W): # goods是物品的集合,W是背包的空闲重量 result = [] sum_weight = 0 ppp = 0 danjia= 0 goods.sort(key=lambda obj: obj.value / obj.weight, reverse=True) for i in goods: if sum_weight + i.weight <= W : sum_weight = sum_weight + i.weight ppp = ppp + i.value danjia = danjia + i.value / i.weight result.append(i.weight) return result, sum_weight, ppp, danjia
这是一个实现背包问题贪心算法的类,其中`greedy`方法接受两个参数,`goods`表示物品的集合,`W`表示背包的剩余空闲重量。函数返回值包含四个部分,`result`表示被选中的物品的重量列表,`sum_weight`表示被选中的物品的总重量,`ppp`表示被选中的物品的总价值,`danjia`表示被选中的物品的平均价值。
在方法中,首先对物品按照单位重量价值从大到小排序,然后依次将物品放入背包中,直到背包装满为止。在放入物品的过程中,记录被选中的物品的重量列表、总重量、总价值和平均价值,并返回这些信息。
阅读全文
相关推荐
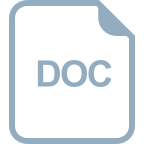
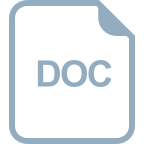
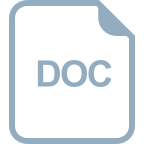
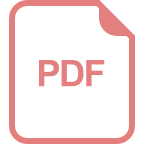
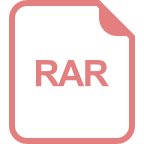
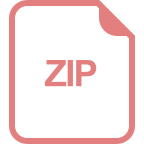
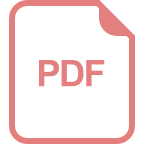
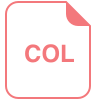
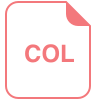
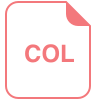
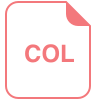
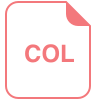
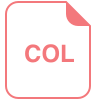
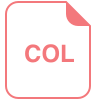
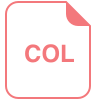
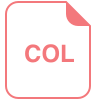
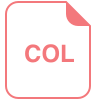
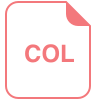