用python做一个动态烟花特效,该界面中上方打开txt文件,获取里面内容并水平滚动显示内容,下方显示烟花特效
时间: 2024-03-22 14:40:12 浏览: 50
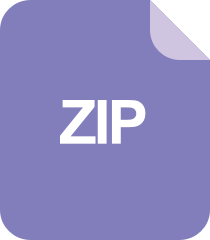
用python实现烟花效果

好的,这里是一个实现动态烟花特效并且可以显示水平滚动文字的Python程序:
```python
import pygame
import random
# 设置窗口大小
WINDOW_WIDTH = 800
WINDOW_HEIGHT = 600
# 设置字体和字体大小
FONT_SIZE = 30
FONT = 'arial.ttf'
# 设置烟花颜色
COLORS = [(255, 255, 255), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (0, 255, 255), (255, 0, 255)]
# 读取文件内容
with open('text.txt', 'r', encoding='utf-8') as f:
content = f.read()
# 初始化pygame
pygame.init()
# 创建窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
# 创建字体对象
font = pygame.font.Font(FONT, FONT_SIZE)
# 计算文本大小
text_width, text_height = font.size(content)
# 创建文本对象
text = font.render(content, True, (255, 255, 255))
# 设置文本位置
text_x = WINDOW_WIDTH
text_y = (WINDOW_HEIGHT - text_height) // 2
# 定义烟花粒子类
class Particle(pygame.sprite.Sprite):
def __init__(self, x, y, color):
super().__init__()
self.color = color
self.image = pygame.Surface((5, 5))
self.image.fill(self.color)
self.rect = self.image.get_rect()
self.rect.center = (x, y)
self.vx = random.randint(-10, 10)
self.vy = random.randint(-20, -10)
self.gravity = 1
def update(self):
self.vx *= 0.98
self.vy += self.gravity
self.rect.x += self.vx
self.rect.y += self.vy
# 创建所有粒子的精灵组
particles = pygame.sprite.Group()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 清空屏幕
window.fill((0, 0, 0))
# 绘制文本
window.blit(text, (text_x, text_y))
# 更新文本位置
text_x -= 2
if text_x < -text_width:
text_x = WINDOW_WIDTH
# 生成烟花
if random.random() < 0.05:
x = random.randint(0, WINDOW_WIDTH)
y = random.randint(WINDOW_HEIGHT // 2, WINDOW_HEIGHT)
color = random.choice(COLORS)
for _ in range(50):
particle = Particle(x, y, color)
particles.add(particle)
# 更新所有烟花粒子
particles.update()
# 绘制所有烟花粒子
particles.draw(window)
# 更新屏幕
pygame.display.update()
```
这个程序中,我们使用了与之前类似的方式来实现水平滚动的文字特效,同时添加了一个烟花特效。在烟花特效中,我们定义了一个`Particle`类来表示烟花粒子,每次循环时随机生成一些粒子,并更新它们的位置和速度。最后,我们将所有粒子添加到一个精灵组中,并在循环中更新和绘制这个精灵组。
你可以根据需要调整窗口大小、字体大小、字体类型以及烟花特效的颜色等参数。
阅读全文
相关推荐
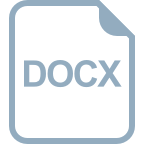
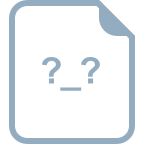
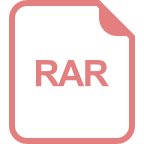
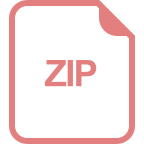
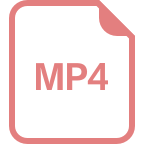
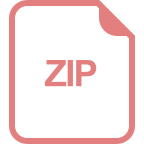
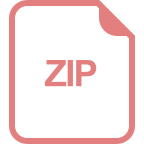
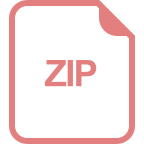