如何使用laspy.read将一个大的文件分割成多个小的文件用numpy缓存读取,并调用matplotlib库生成可视化结果
时间: 2023-06-08 11:07:35 浏览: 63
您可以按照以下步骤将大的LAS文件拆分成多个小的文件并使用numpy缓存读取:
1. 使用laspy库中的laspy.file.File()方法打开LAS文件。
2. 获取LAS文件总点数(点的数量)以及每个小文件中的点数。
3. 使用numpy库中的arange()方法和linspace()方法来确定生成每个小文件的范围。
4. 使用laspy库中的fileWriter()方法将LAS文件中的每个点写入到小文件中。
5. 使用numpy库中的load()方法加载所有小文件,并将它们存储在一个numpy数组中。
6. 使用matplotlib库中的plot()方法生成可视化结果。
具体代码实现可以参考以下示例:
```Python
import laspy
import numpy as np
import matplotlib.pylab as plt
# 设置LAS文件名和小文件点数
las_filename = "file.las"
batch_size = 1000000
# 打开LAS文件并获取总点数
f = laspy.file.File(las_filename, mode='r')
total_points = f.header.point_count
# 获取每个小文件的点数范围
indices = np.arange(0, total_points, batch_size)
ranges = np.linspace(0, total_points, len(indices) + 1, dtype=int)
# 将LAS文件拆分为小文件并使用numpy缓存读取
for index, start, end in zip(indices, ranges[:-1], ranges[1:]):
with laspy.file.File('small_file_{}.las'.format(index), mode='w', header=f.header) as outfile:
points = f.points[start:end]
outfile.points = points
outfile.close()
data = np.load('small_file_{}.las.npy'.format(index))
print('Loaded {} in memory'.format(data.shape))
# 使用matplotlib库生成可视化结果
dataset = np.concatenate([np.load('small_file_{}.las.npy'.format(index)) for index, start, end in zip(indices, ranges[:-1], ranges[1:])], axis=0)
plt.scatter(dataset['X'], dataset['Y'], s=0.1, c=dataset['Z'])
plt.show()
```
此示例代码将LAS文件分割成小文件,并使用numpy缓存读取以提高性能,并使用matplotlib库生成可视化结果。您可以根据需要自定义LAS文件名和小文件点数,并通过更改参数中的s值和c值来调整散点图的大小和颜色。
相关推荐
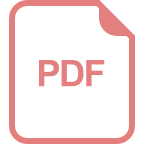
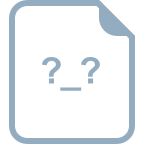















