python中判断字符串是否以你好开头
时间: 2023-10-18 17:14:03 浏览: 42
可以使用字符串的 `startswith()` 方法来判断一个字符串是否以另一个字符串开头,示例代码如下:
```python
s = "你好,世界!"
if s.startswith("你好"):
print("以你好开头")
else:
print("不以你好开头")
```
输出结果为:
```
以你好开头
```
相关问题
python判断字符串是否只含数字、中文、英文、下划线
可以使用正则表达式来判断字符串是否只含有数字、中文、英文、下划线,具体实现如下:
```python
import re
def is_valid_string(string):
# 匹配只含有数字、中文、英文、下划线的正则表达式
pattern = re.compile(r'^[0-9a-zA-Z_\u4e00-\u9fa5]+$')
return pattern.match(string) is not None
```
使用示例:
```python
print(is_valid_string("hello_world123")) # True
print(is_valid_string("你好世界")) # True
print(is_valid_string("hello, world!")) # False
```
其中,正则表达式 `^[0-9a-zA-Z_\u4e00-\u9fa5]+$` 中:
- `^` 表示匹配字符串的开头;
- `$` 表示匹配字符串的结尾;
- `[0-9a-zA-Z_]` 表示匹配数字、字母和下划线;
- `[\u4e00-\u9fa5]` 表示匹配中文字符。
list = ['sg.bigo.live','GLThread 2646','GLThread 2639', 'BigoAudioDevMan', 'Thread-870', 'encode_thread']python匹配list中以字符串‘GLT’开头的字符
### 回答1:
串,该如何实现?
你好!关于你的问题,可以使用Python的字符串方法startswith()进行匹配。具体实现代码如下所示:
```
list = ['sg.bigo.live', 'GLThread 2646', 'GLThread 2639', 'BigoAudioDevMan', 'Thread-870', 'encode_thread']
result = [s for s in list if s.startswith('GLT')]
print(result)
```
运行结果如下所示:
```
['GLThread 2646', 'GLThread 2639']
```
这样就可以匹配出所有以字符串‘GLT’开头的字符串了。希望能够帮到你!
### 回答2:
可以使用Python的列表推导式来匹配list中以字符串'GLT'开头的字符。具体实现如下:
```python
list = ['sg.bigo.live','GLThread 2646','GLThread 2639', 'BigoAudioDevMan', 'Thread-870', 'encode_thread']
matched_strings = [string for string in list if string.startswith('GLT')]
```
上述代码中,通过列表推导式对list中的每个字符串进行遍历,如果字符串以'GLT'开头,则将其添加到新的列表matched_strings中。最终,matched_strings中存储的就是以字符串'GLT'开头的字符。
注意,列表推导式可以简洁地实现匹配操作,但也可以使用传统的for循环遍历list并进行匹配。
### 回答3:
可以使用列表推导式来实现匹配以字符串'GLT'开头的字符。代码如下:
```python
list = ['sg.bigo.live','GLThread 2646','GLThread 2639', 'BigoAudioDevMan', 'Thread-870', 'encode_thread']
result = [string for string in list if string.startswith('GLT')]
print(result)
```
运行结果为:
```
['GLThread 2646', 'GLThread 2639']
```
解释:列表推导式 `[string for string in list if string.startswith('GLT')]` 遍历了列表中的每个元素,判断每个元素是否以字符串'GLT'开头,如果是,则将其添加到结果列表中。最后打印结果列表,即可得到匹配结果。
相关推荐
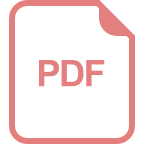









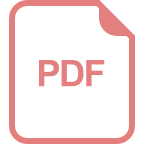
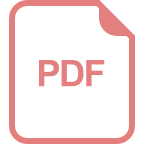
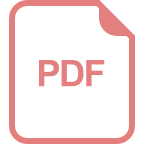