ts 父类调用子类方法
时间: 2023-08-18 18:39:18 浏览: 98
在 TypeScript 中,父类可以通过声明一个子类的实例来调用子类的方法。具体实现如下:
```typescript
class Parent {
constructor() {}
callChildMethod(child: Child) {
child.childMethod();
}
}
class Child extends Parent {
constructor() {
super();
}
childMethod() {
console.log("This is a child method.");
}
}
const child = new Child();
const parent = new Parent();
parent.callChildMethod(child); // 输出 "This is a child method."
```
在上面的代码中,`Parent` 类中的 `callChildMethod` 方法接收一个 `Child` 类的实例作为参数,并调用该实例的 `childMethod` 方法。在 `Child` 类中,`childMethod` 方法被实现并输出一段文字。当我们创建一个 `Child` 类的实例 `child` 和一个 `Parent` 类的实例 `parent` 后,我们可以通过调用 `parent.callChildMethod(child)` 方法来调用 `Child` 类中的 `childMethod` 方法。
相关问题
vuets子调用父组件方法
在Vue中,子组件可以通过props接收父组件传递的方法,并在子组件中调用该方法。在Vue中使用TypeScript时,我们可以使用类似于以下的方式来实现子组件调用父组件方法:
1. 在父组件中定义一个方法,并将该方法作为属性传递给子组件:
```typescript
// 父组件
<template>
<div>
<Child :parentMethod="parentMethod"></Child>
</div>
</template>
<script lang="ts">
import { Vue, Component } from 'vue-property-decorator';
import Child from './Child.vue';
@Component({
components: {
Child,
},
})
export default class Parent extends Vue {
parentMethod() {
console.log('这是父组件中的方法');
}
}
</script>
```
Android webview调用react ts方法
您好,我可以回答这个问题。在 Android WebView 中调用 React TS 方法,您需要先在 React TS 代码中将需要调用的方法暴露出来,然后在 WebView 中使用 JavaScriptInterface 注解将该方法暴露给 WebView,最后在 WebView 中使用 JavaScript 调用该方法即可。具体实现可以参考相关文档和示例代码。
相关推荐
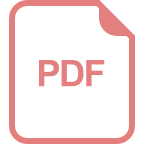
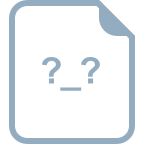
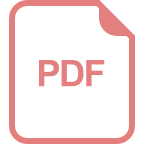











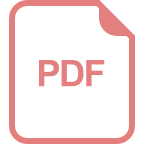