用c#写一个五子棋程序
时间: 2023-10-19 08:07:44 浏览: 91
以下是一个简单的五子棋程序的C#代码示例:
```csharp
using System;
namespace FiveInARow
{
class Program
{
static int ROWS = 15;
static int COLS = 15;
static char[,] board = new char[ROWS, COLS];
static void Main(string[] args)
{
InitializeBoard();
PrintBoard();
char player = 'X';
bool gameOver = false;
while (!gameOver)
{
Console.WriteLine("Player " + player + "'s turn:");
Console.Write("Enter row number (1-" + ROWS + "): ");
int row = Convert.ToInt32(Console.ReadLine()) - 1;
Console.Write("Enter column number (1-" + COLS + "): ");
int col = Convert.ToInt32(Console.ReadLine()) - 1;
if (IsValidMove(row, col))
{
PlacePiece(row, col, player);
if (IsWinningMove(row, col))
{
Console.WriteLine("Player " + player + " wins!");
gameOver = true;
}
else if (IsBoardFull())
{
Console.WriteLine("It's a tie!");
gameOver = true;
}
else
{
player = (player == 'X') ? 'O' : 'X';
PrintBoard();
}
}
else
{
Console.WriteLine("Invalid move. Please try again.");
}
}
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
}
static void InitializeBoard()
{
for (int row = 0; row < ROWS; row++)
{
for (int col = 0; col < COLS; col++)
{
board[row, col] = '-';
}
}
}
static void PrintBoard()
{
Console.WriteLine();
for (int row = 0; row < ROWS; row++)
{
for (int col = 0; col < COLS; col++)
{
Console.Write(board[row, col] + " ");
}
Console.WriteLine();
}
Console.WriteLine();
}
static bool IsValidMove(int row, int col)
{
if (row < 0 || row >= ROWS || col < 0 || col >= COLS)
{
return false;
}
return board[row, col] == '-';
}
static void PlacePiece(int row, int col, char player)
{
board[row, col] = player;
}
static bool IsWinningMove(int row, int col)
{
char player = board[row, col];
// check horizontal
int count = 1;
int c = col - 1;
while (c >= 0 && board[row, c] == player)
{
count++;
c--;
}
c = col + 1;
while (c < COLS && board[row, c] == player)
{
count++;
c++;
}
if (count >= 5)
{
return true;
}
// check vertical
count = 1;
int r = row - 1;
while (r >= 0 && board[r, col] == player)
{
count++;
r--;
}
r = row + 1;
while (r < ROWS && board[r, col] == player)
{
count++;
r++;
}
if (count >= 5)
{
return true;
}
// check diagonal 1
count = 1;
r = row - 1;
c = col - 1;
while (r >= 0 && c >= 0 && board[r, c] == player)
{
count++;
r--;
c--;
}
r = row + 1;
c = col + 1;
while (r < ROWS && c < COLS && board[r, c] == player)
{
count++;
r++;
c++;
}
if (count >= 5)
{
return true;
}
// check diagonal 2
count = 1;
r = row - 1;
c = col + 1;
while (r >= 0 && c < COLS && board[r, c] == player)
{
count++;
r--;
c++;
}
r = row + 1;
c = col - 1;
while (r < ROWS && c >= 0 && board[r, c] == player)
{
count++;
r++;
c--;
}
if (count >= 5)
{
return true;
}
return false;
}
static bool IsBoardFull()
{
for (int row = 0; row < ROWS; row++)
{
for (int col = 0; col < COLS; col++)
{
if (board[row, col] == '-')
{
return false;
}
}
}
return true;
}
}
}
```
这个程序使用一个二维字符数组来表示棋盘,使用 `-` 表示空位置,使用 `X` 和 `O` 表示两个玩家。程序使用一个循环来不断让两个玩家交替下棋,直到出现胜利或平局为止。在每个回合中,程序会让当前玩家输入一个位置来下棋,然后检查该位置是否有效,如果是,就下棋并检查是否出现胜利或平局。如果没有出现胜利或平局,则切换到另一个玩家,然后打印棋盘以供下一个回合使用。如果出现胜利或平局,程序将退出循环并显示相应的消息。
阅读全文
相关推荐





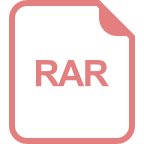

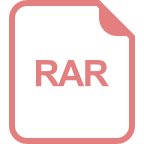




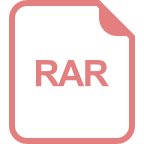

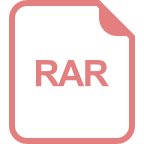
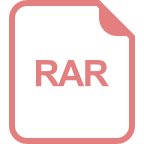


