小明写了一段代码,想实现替换文件部分内容的目标,但是代码似乎写得有些问题,请你纠正并优化这段代码,并给出你的优化逻辑,优化点多多益善。 public static void main(String[] args) throws IOException { File file = new File("some file path"); List list1 = new ArrayList(); FileInputStream fileInputStream = new FileInputStream(file); byte[] bytes = new byte[1024]; int i = fileInputStream.read(bytes); if(i > 0) { do { i = fileInputStream.read(bytes); String s = new String(bytes, 0 , i); list1.add(s); } while(i != -1); } i = fileInputStream.read(bytes); String start = new String(bytes, 0, i); list1.add(start); for (int i1 = 0; i1 < list1.size(); i1++) { String s = (String) list1.get(i1); if(s.contains("1")) { s = s.replace("1", "a"); } else if(s.contains("2")) { s = s.replace("2", "b"); } else if(s.contains("3")) { s = s.replace("3", "c"); } else if(s.contains("4")) { s = s.replace("4", "d"); } list1.remove(i1); list1.add(i1, s); } FileOutputStream fos = new FileOutputStream(file); for (Object o : list1) { fos.write(o.toString().getBytes()); } }
时间: 2023-05-31 12:04:43 浏览: 101
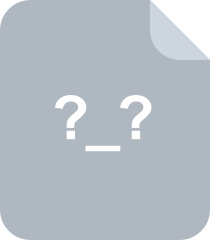
替换文件的代码
public static void main(String[] args) throws IOException {
File file = new File("some file path");
List<String> list1 = new ArrayList<>(); // 使用泛型
FileInputStream fileInputStream = new FileInputStream(file);
int i = 0;
byte[] bytes = new byte[1024];
while ((i = fileInputStream.read(bytes)) != -1) { // 使用while循环读取文件
String s = new String(bytes, 0, i);
list1.add(s);
}
fileInputStream.close(); // 关闭输入流
String start = list1.get(0); // 第一项即为文件内容
list1.remove(0); // 移除第一项
for (int i1 = 0; i1 < list1.size(); i1++) { // 循环遍历list1
String s = list1.get(i1);
if (s.contains("1")) {
s = s.replace("1", "a");
} else if (s.contains("2")) {
s = s.replace("2", "b");
} else if (s.contains("3")) {
s = s.replace("3", "c");
} else if (s.contains("4")) {
s = s.replace("4", "d");
}
list1.set(i1, s); // 直接使用set方法替换原来的元素
}
FileOutputStream fos = new FileOutputStream(file);
fos.write(start.getBytes()); // 先写入原来的文件内容
for (String s : list1) { // 循环遍历list1写入修改后的内容
fos.write(s.getBytes());
}
fos.close(); // 关闭输出流
}
优化逻辑:
1. 使用泛型,避免了类型转换;
2. 使用while循环读取文件,避免了重复读取;
3. 关闭输入流和输出流,避免资源泄漏;
4. 直接使用set方法替换原来的元素,避免了移除和添加的操作;
5. 先写入原来的文件内容,再写入修改后的内容,避免了覆盖原来的文件内容。
阅读全文
相关推荐
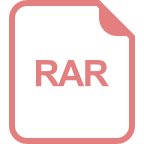
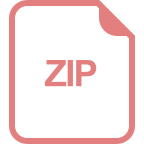
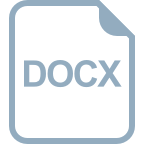
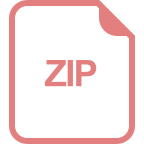
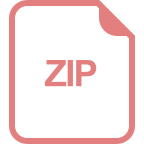
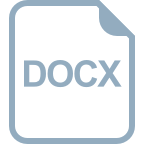
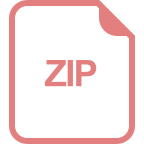
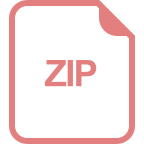
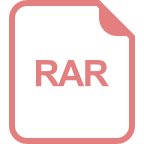
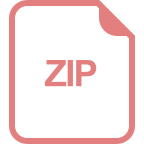
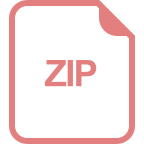
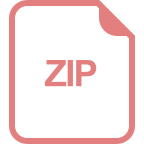
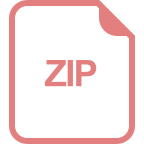