c/c++提取TXT文本中序号,x,y,z坐标信息中间逗号隔开
时间: 2023-08-31 11:18:37 浏览: 175
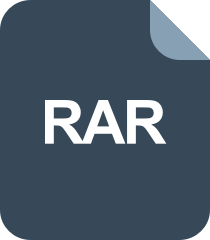
txt文件读取以逗号为分隔符号

### 回答1:
可以使用C/C++中的字符串处理函数和流处理器来实现对TXT文本中序号,x,y,z坐标信息的提取。可以先读取每一行文本数据,然后使用字符串处理函数(如strtok、substr等)和流处理器(如istringstream)逐个提取出每个数据信息,再进行处理和存储。需要注意的是,提取出的数据信息可能需要进行类型转换和错误处理。
### 回答2:
要提取TXT文本中的序号、x、y、z坐标信息,可以使用C/C++编程语言进行操作。首先,我们需要读取TXT文件并逐行进行处理。下面是一个可能的实现解答:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <sstream>
using namespace std;
struct Coordinate {
int index;
float x, y, z;
};
vector<Coordinate> extractCoordinates(string filename) {
vector<Coordinate> coordinates;
// 读取TXT文件
ifstream file(filename);
if (!file) {
cerr << "无法打开文件" << filename << endl;
return coordinates;
}
string line;
while (getline(file, line)) {
stringstream ss(line);
Coordinate coord;
string token;
// 使用逗号分割每行中的数据
getline(ss, token, ',');
coord.index = stoi(token);
getline(ss, token, ',');
coord.x = stof(token);
getline(ss, token, ',');
coord.y = stof(token);
getline(ss, token, ',');
coord.z = stof(token);
coordinates.push_back(coord);
}
file.close();
return coordinates;
}
int main() {
string filename = "coordinates.txt";
vector<Coordinate> coordinates = extractCoordinates(filename);
// 输出提取的坐标信息
for (const auto& coord : coordinates) {
cout << "序号: " << coord.index << ", X: " << coord.x << ", Y: " << coord.y << ", Z: " << coord.z << endl;
}
return 0;
}
```
以上代码首先定义了一个名为`Coordinate`的结构体,用于存储序号、x、y、z坐标信息。接着,`extractCoordinates`函数会接收一个TXT文件的文件名作为参数,并返回一个存储了提取的坐标信息的向量。在该函数中,我们先打开文件,逐行读取文件内容,并使用`getline`函数和逗号作为分隔符来提取序号、x、y、z坐标信息,并将其存储到一个`Coordinate`结构体变量中,然后将该结构体变量添加到一个向量中作为结果。最后,在`main`函数中调用`extractCoordinates`函数来提取TXT文件中的坐标信息,并进行输出。
注意:以上代码仅用于提供一个可能的实现解答,具体实现可能因编译环境或其他因素而有所调整。
### 回答3:
可以使用C语言来提取TXT文本中序号、x、y、z坐标信息,这些信息之间是逗号隔开的。首先,我们需要打开TXT文件并读取其中的内容。然后,我们可以使用字符串处理函数来从每一行中提取出需要的信息。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
FILE *file = fopen("data.txt", "r"); // 打开文件(假设文件名为data.txt)
char line[100]; // 用于存储每一行的字符串
char *token; // 用于存储每个字段的指针
if (file == NULL) { // 检查文件是否成功打开
printf("无法打开文件!\n");
return 1;
}
while (fgets(line, sizeof(line), file)) { // 逐行读取文件内容
token = strtok(line, ","); // 使用逗号作为分隔符
// 提取序号信息
printf("序号:%s\n", token);
// 提取x坐标信息
token = strtok(NULL, ",");
printf("x坐标:%s\n", token);
// 提取y坐标信息
token = strtok(NULL, ",");
printf("y坐标:%s\n", token);
// 提取z坐标信息
token = strtok(NULL, ",");
printf("z坐标:%s\n", token);
}
fclose(file); // 关闭文件
return 0;
}
```
假设data.txt文件的内容如下:
```
1,10,20,30
2,15,25,35
3,22,33,44
```
运行上述代码后,将会输出:
```
序号:1
x坐标:10
y坐标:20
z坐标:30
序号:2
x坐标:15
y坐标:25
z坐标:35
序号:3
x坐标:22
y坐标:33
z坐标:44
```
以上代码演示了如何使用C语言从TXT文本中提取序号、x、y、z坐标信息,并使用逗号进行分隔。请根据实际情况进行适当修改。
阅读全文
相关推荐
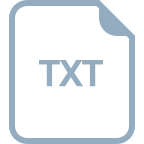
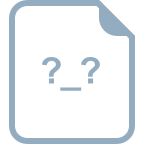
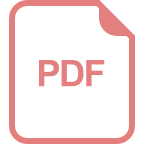
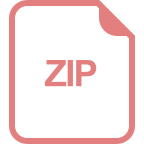
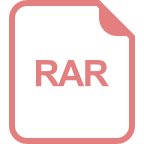
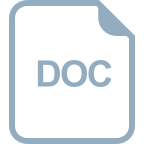
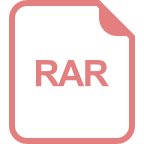
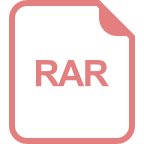
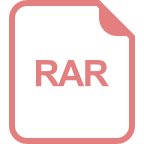
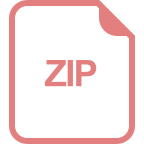
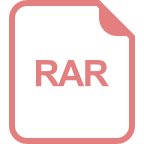
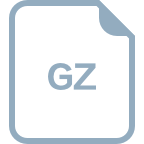
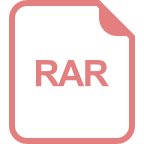