/*! jQuery jquery.com | jquery.org/license */ (function(e,t){function _(e){var t=M[e]={};return v.each(e.split(y),function(e,n){t[n]=!0}),t}function H(e,n,r){if(r===t&&e.nodeType===1){var i="data-" n.replace(P,"-$1").toLowerCase();r=e.getAttribute(i);if(typeof r=="string"){try{r=r==="true"?!0:r==="false"?!1:r==="null"?null: r ""===r? r:D.test(r)?v.parseJSON(r):r}catch(s){}v.data(e,n,r)}else r=t}return r}function B(e){var t;for(t in e){if(t==="data"&&v.isEmptyObject(e[t]))continue;if(t!=="toJSON")return!1}return!0}function et(){return!1}function tt(){return!0}function ut(e){return!e||!e.parentNode||e.parentNode.nodeType===11}function at(e,t){do e=e[t];while(e&&e.nodeType!==1);return e}function ft(e,t,n){t=t||0;if(v.isFunction(t))return v.grep(e,function(e,r){var i=!!t.call(e,r,e);return i===n});if(t.nodeType)return v.grep(e,function(e,r){return e===t===n});if(typeof t=="string"){var r=v.grep(e,function(e){return e.nodeType===1});if(it.test(t))return v.filter(t,r,!n);t=v.filter(t,r)}return v.grep(e,function(e,r){return v.inArray(e,t)>=0===n})}function lt(e){var t=ct.split("|"),n=e.createDocumentFragment();if(n.createElement)while(t.length)n.createElement(t.pop());return n}function Lt(e,t){return e.getElementsByTagName(t)[0]||e.appendChild(e.ownerDocument.createElement(t))}function At(e,t){if(t.nodeType!==1||!v.hasData(e))return;var n,r,i,s=v._data(e),o=v._data(t,s),u=s.events;if(u){delete o.handle,o.events={};for(n in u)for(r=0,i=u[n].length;r<i;r )v.event.add(t,n,u[n][r])}o.data&&(o.data=v.extend({},o.data))}function Ot(e,t){
时间: 2024-04-10 07:31:43 浏览: 122
这段代码是 jQuery 库的一部分,用于处理 DOM 元素和数据的操作。它定义了一些常用的工具函数和方法,以及事件处理和数据缓存的功能。
具体来说,这段代码包含了一些常用的工具函数,例如 `isFunction`、`isEmptyObject`、`inArray` 等。还有一些事件处理相关的函数,例如 `event.add`、`event.remove` 等。此外,还有一些用于操作 DOM 元素和数据缓存的函数,例如 `data`、`attr`、`prop` 等。
这段代码还包含了一些用于处理兼容性和性能优化的技巧,例如使用文档片段创建元素、使用原生的 `getElementById` 方法等。
总而言之,这段代码提供了一些常用的功能和工具,可以简化开发者在操作 DOM 元素和处理数据时的代码编写。
相关问题
这段代码改成可调用函数 : /*! * ASP.NET SignalR JavaScript Library 2.4.3 * http://signalr.net/ * * Copyright (c) .NET Foundation. All rights reserved. * Licensed under the Apache License, Version 2.0. See License.txt in the project root for license information. * */ /// <reference path="....\SignalR.Client.JS\Scripts\jquery-1.6.4.js" /> /// <reference path="jquery.signalR.js" /> (function ($, window, undefined) { /// <param name="$" type="jQuery" /> "use strict"; if (typeof ($.signalR) !== "function") { throw new Error("SignalR: SignalR is not loaded. Please ensure jquery.signalR-x.js is referenced before ~/signalr/js."); } var signalR = $.signalR; function makeProxyCallback(hub, callback) { return function () { // Call the client hub method callback.apply(hub, $.makeArray(arguments)); }; } function registerHubProxies(instance, shouldSubscribe) { var key, hub, memberKey, memberValue, subscriptionMethod; for (key in instance) { if (instance.hasOwnProperty(key)) { hub = instance[key]; if (!(hub.hubName)) { // Not a client hub continue; } if (shouldSubscribe) { // We want to subscribe to the hub events subscriptionMethod = hub.on; } else { // We want to unsubscribe from the hub events subscriptionMethod = hub.off; } // Loop through all members on the hub and find client hub functions to subscribe/unsubscribe for (memberKey in hub.client) { if (hub.client.hasOwnProperty(memberKey)) { memberValue = hub.client[memberKey]; if (!$.isFunction(memberValue)) { // Not a client hub function continue; } // Use the actual user-provided callback as the "identity" value for the registration. subscriptionMethod.call(hub, memberKey, makeProxyCallback(hub, memberValue), memberValue); } } } } } $.hubConnection.prototype.createHubProxies = function () { var proxies = {}; this.starting(function () { // Register the hub proxies as subscribed // (instance, shouldSubscribe) registerHubProxies(proxies, true); this._registerSubscribedHubs(); }).disconnected(function () { // Unsubscribe all hub proxies when we "disconnect". This is to ensure that we do not re-add functional call backs. // (instance, shouldSubscribe) registerHubProxies(proxies, false); }); proxies['realDataHub'] = this.createHubProxy('realDataHub'); proxies['realDataHub'].client = { }; proxies['realDataHub'].server = { send: function (name, message) { return proxies['realDataHub'].invoke.apply(proxies['realDataHub'], $.merge(["Send"], $.makeArray(arguments))); } }; return proxies; }; signalR.hub = $.hubConnection("/signalr", { useDefaultPath: false }); $.extend(signalR, signalR.hub.createHubProxies()); }(window.jQuery, window));
您可以将上述代码封装成一个可调用函数,例如:
```javascript
function connectToHub() {
if (typeof ($.signalR) !== "function") {
throw new Error("SignalR: SignalR is not loaded. Please ensure jquery.signalR-x.js is referenced before ~/signalr/js.");
}
var signalR = $.signalR;
var hubConnection = signalR.hubConnection("/signalr", { useDefaultPath: false });
var proxies = {};
function makeProxyCallback(hub, callback) {
return function () {
callback.apply(hub, $.makeArray(arguments));
};
}
function registerHubProxies(instance, shouldSubscribe) {
var key, hub, memberKey, memberValue, subscriptionMethod;
for (key in instance) {
if (instance.hasOwnProperty(key)) {
hub = instance[key];
if (!(hub.hubName)) {
continue;
}
if (shouldSubscribe) {
subscriptionMethod = hub.on;
} else {
subscriptionMethod = hub.off;
}
for (memberKey in hub.client) {
if (hub.client.hasOwnProperty(memberKey)) {
memberValue = hub.client[memberKey];
if (!$.isFunction(memberValue)) {
continue;
}
subscriptionMethod.call(hub, memberKey, makeProxyCallback(hub, memberValue), memberValue);
}
}
}
}
}
hubConnection.starting(function () {
registerHubProxies(proxies, true);
this._registerSubscribedHubs();
}).disconnected(function () {
registerHubProxies(proxies, false);
});
proxies['realDataHub'] = hubConnection.createHubProxy('realDataHub');
proxies['realDataHub'].client = {};
proxies['realDataHub'].server = {
send: function (name, message) {
return proxies['realDataHub'].invoke.apply(proxies['realDataHub'], $.merge(["Send"], $.makeArray(arguments)));
}
};
return proxies;
}
```
然后您可以在需要连接 SignalR 的地方调用该函数,例如:
```javascript
var hubProxies = connectToHub();
hubProxies.realDataHub.server.send('John', 'Hello!');
```
阅读全文
相关推荐




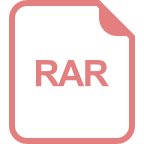



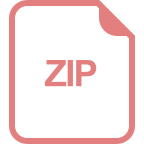








