利用C语言实现二分查找算法。
时间: 2024-02-29 15:51:19 浏览: 28
好的,以下是一个简单的C语言代码实现二分查找算法的例子:
```c
#include <stdio.h>
int binary_search(int arr[], int len, int target) {
int low = 0;
int high = len - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1;
}
int main() {
int arr[] = {1, 3, 5, 7, 9, 11};
int len = sizeof(arr) / sizeof(arr[0]);
int target = 7;
int index = binary_search(arr, len, target);
if (index == -1) {
printf("The target is not found in the array.\n");
} else {
printf("The target is found at index %d.\n", index);
}
return 0;
}
```
该程序首先定义一个 `binary_search` 函数,接受一个已排序的数组、数组长度和一个目标值作为参数,返回目标值在数组中的索引。如果目标值不在数组中,返回 -1。
接下来,在 `main` 函数中,我们定义一个已排序的数组 `arr`,数组长度 `len` 和目标值 `target`。然后,我们调用 `binary_search` 函数来查找目标值在数组中的位置,并打印出结果。
注意,这个例子假设数组已经按升序排列。如果数组是按降序排列的,只需要将 `if` 和 `else` 语句块的条件判断反过来即可。
相关推荐
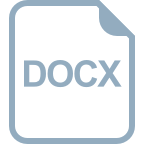














