调用PCL点云库写一段点云分割的算法
时间: 2024-05-14 14:12:12 浏览: 10
以下是使用PCL点云库实现基于欧几里得聚类的点云分割算法示例代码:
```c++
#include <iostream>
#include <pcl/point_types.h>
#include <pcl/filters/extract_indices.h>
#include <pcl/segmentation/extract_clusters.h>
#include <pcl/kdtree/kdtree.h>
int main(int argc, char** argv)
{
// Load point cloud data
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::io::loadPCDFile("input_cloud.pcd", *cloud);
// Create KDTree for efficient nearest neighbor search
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>);
tree->setInputCloud(cloud);
// Create vector for storing cluster indices
std::vector<pcl::PointIndices> cluster_indices;
// Create EuclideanClusterExtraction object and set parameters
pcl::EuclideanClusterExtraction<pcl::PointXYZ> ec;
ec.setClusterTolerance(0.02f); // Maximum distance between points in a cluster
ec.setMinClusterSize(100); // Minimum number of points in a cluster
ec.setMaxClusterSize(25000); // Maximum number of points in a cluster
ec.setSearchMethod(tree);
ec.setInputCloud(cloud);
// Extract clusters
ec.extract(cluster_indices);
// Print number of clusters found
std::cout << "Number of clusters found: " << cluster_indices.size() << std::endl;
// Create ExtractIndices object for each cluster and save to file
for (int i = 0; i < cluster_indices.size(); ++i)
{
pcl::ExtractIndices<pcl::PointXYZ> extract;
extract.setInputCloud(cloud);
extract.setIndices(boost::make_shared<const pcl::PointIndices>(cluster_indices[i]));
pcl::PointCloud<pcl::PointXYZ>::Ptr cluster(new pcl::PointCloud<pcl::PointXYZ>);
extract.filter(*cluster);
pcl::io::savePCDFile("cluster_" + std::to_string(i) + ".pcd", *cluster);
}
return 0;
}
```
此代码将从名为“input_cloud.pcd”的文件中加载点云数据,使用KDTree进行最近邻搜索并执行欧几里得聚类,最后将每个簇的点保存到单独的PCD文件中。聚类的参数(聚类容差、最小/最大簇大小等)可以根据应用程序的需要进行调整。
相关推荐
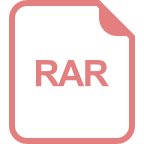














