VHDL交通灯控制器设计
时间: 2023-08-08 08:08:42 浏览: 58
交通灯控制器可以使用VHDL来实现。以下是一个简单的交通灯控制器的VHDL代码实现:
```
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity traffic_light is
port (
clk : in std_logic;
reset : in std_logic;
green : out std_logic;
yellow : out std_logic;
red : out std_logic
);
end entity traffic_light;
architecture behavioral of traffic_light is
type state_type is (green_state, yellow_state, red_state);
signal state : state_type := green_state;
constant green_time : integer := 10000; -- 10 seconds
constant yellow_time : integer := 2000; -- 2 seconds
constant red_time : integer := 10000; -- 10 seconds
signal counter : unsigned(14 downto 0) := (others => '0');
begin
process (clk, reset)
begin
if reset = '1' then
state <= green_state;
counter <= (others => '0');
elsif rising_edge(clk) then
case state is
when green_state =>
green <= '1';
yellow <= '0';
red <= '0';
if counter = green_time then
state <= yellow_state;
counter <= (others => '0');
else
counter <= counter + 1;
end if;
when yellow_state =>
green <= '0';
yellow <= '1';
red <= '0';
if counter = yellow_time then
state <= red_state;
counter <= (others => '0');
else
counter <= counter + 1;
end if;
when red_state =>
green <= '0';
yellow <= '0';
red <= '1';
if counter = red_time then
state <= green_state;
counter <= (others => '0');
else
counter <= counter + 1;
end if;
end case;
end if;
end process;
end architecture behavioral;
```
在代码中,我们定义了一个状态机来控制交通灯的状态。在每个状态下,我们使用计数器来计算状态的持续时间。使用计数器是为了定时控制交通灯的切换。当计数器到达指定的时间时,状态机会将交通灯的状态切换到下一个状态,并将计数器重置为零。
在主过程中,我们使用了一个 `process` 来控制状态机。如果 `reset` 信号为高电平,状态机将被重置为 `green_state` ,并将计数器清零。在上升沿时,状态机进入当前状态,并根据当前状态设置交通灯的输出信号。如果计数器达到指定时间,状态机将切换到下一个状态,并将计数器重置为零。
注意,这里使用了 `ieee.numeric_std.all` 库,以便使用无符号整数类型 `unsigned`。这个库是标准VHDL库的一部分,可以用于处理数字类型的操作。
以上就是一个简单的交通灯控制器的VHDL代码实现。
相关推荐
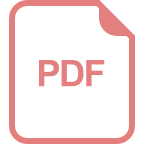
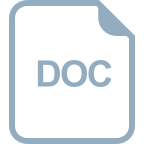
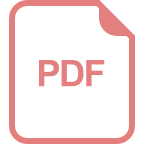
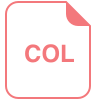













