java hutool bean list 转map
时间: 2023-07-04 19:08:48 浏览: 332
可以使用 Hutool 工具包中的 `BeanUtil` 类来将一个 List 转换为一个 Map。
示例代码如下:
```java
import cn.hutool.core.bean.BeanUtil;
import cn.hutool.core.collection.CollUtil;
import java.util.List;
import java.util.Map;
public class Test {
public static void main(String[] args) {
// 假设有一个类 User,有属性 id、name、age
List<User> userList = CollUtil.newArrayList(
new User(1L, "张三", 20),
new User(2L, "李四", 21),
new User(3L, "王五", 22)
);
// 将 List 转为 Map,以 id 为 key,User 对象为 value
Map<Long, User> userMap = CollUtil.toMap(userList, user -> user.getId(), user -> user);
// 打印转换后的 Map
System.out.println(userMap);
}
static class User {
private Long id;
private String name;
private Integer age;
public User(Long id, String name, Integer age) {
this.id = id;
this.name = name;
this.age = age;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
'}';
}
}
}
```
在上面的示例代码中,我们使用 `CollUtil.toMap()` 方法将 List 转为 Map,其中第一个参数是要转换的 List,第二个参数是 key 的获取方式,第三个参数是 value 的获取方式。在本例中,我们将 id 作为 key,User 对象作为 value。
阅读全文
相关推荐
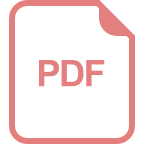
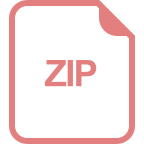










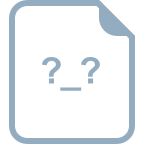
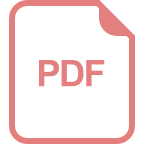
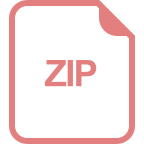
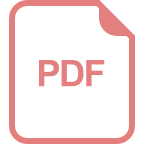
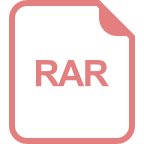