python使用cv2实现Guo-Hall算法
时间: 2024-03-16 19:45:52 浏览: 65
Guo-Hall算法是一种边缘细化算法,可以通过CV2库中的函数实现。下面是使用Python和CV2库实现Guo-Hall算法的示例代码:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('input_image.png', cv2.IMREAD_GRAYSCALE)
# 二值化图像
ret, img = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY)
# Guo-Hall算法
def guo_hall(img):
h, w = img.shape
dst = np.zeros((h, w), np.uint8)
for i in range(h):
for j in range(w):
if img[i, j] == 0: # 黑点
p2 = img[i-1, j] if i > 0 else 0
p3 = img[i-1, j+1] if i > 0 and j < w-1 else 0
p4 = img[i, j+1] if j < w-1 else 0
p5 = img[i+1, j+1] if i < h-1 and j < w-1 else 0
p6 = img[i+1, j] if i < h-1 else 0
p7 = img[i+1, j-1] if i < h-1 and j > 0 else 0
p8 = img[i, j-1] if j > 0 else 0
p9 = img[i-1, j-1] if i > 0 and j > 0 else 0
# 计算C(p1)值
cp1 = ((p2 == 0 and p3 == 1) + (p3 == 0 and p4 == 1) +
(p4 == 0 and p5 == 1) + (p5 == 0 and p6 == 1) +
(p6 == 0 and p7 == 1) + (p7 == 0 and p8 == 1) +
(p8 == 0 and p9 == 1) + (p9 == 0 and p2 == 1))
# 计算N(p1)值
np1 = p2 + p3 + p4 + p5 + p6 + p7 + p8 + p9
# 判断是否为端点
if np1 >= 2 and np1 <= 6 and cp1 == 1:
if p2 * p4 * p6 == 0 and p4 * p6 * p8 == 0:
dst[i, j] = 255
return dst
# 细化图像
img_thin = guo_hall(img)
# 显示图像
cv2.imshow('input', img)
cv2.imshow('thin', img_thin)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们首先读取输入图像,然后将其转换为二值图像。接下来,我们实现了Guo-Hall算法,该算法使用8邻域像素值来计算C(p1)和N(p1)值,并根据它们的值判断是否为端点。最后,我们应用Guo-Hall算法来细化输入图像,并显示输入和细化图像。
相关推荐
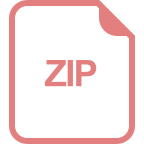
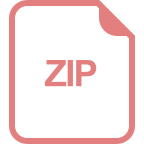
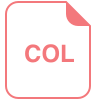
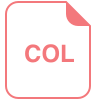
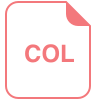
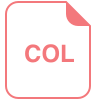
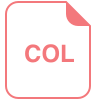









