c++编写一个程序,定义一个类student,数据成员包含:num(学号),name(姓名),score(成绩),count(人数),sum(总分)。 要求: 1、定义count、sum为静态数据成员。 2、定义有参构造函数。 3、定义void show()函数。//输出:输出序号,姓名,成绩 4、定义静态成员函数 void show_count_sum(。//输出:人数,总分 5、在main中定义三个对象,分别调用void show()和 void show_count_sum(),并观察运行结果。
时间: 2024-03-10 11:49:55 浏览: 19
以下是你需要的程序:
```c++
#include <iostream>
#include <string>
using namespace std;
class student {
private:
int num;
string name;
float score;
static int count;
static float sum;
public:
student(int n, string na, float s) {
num = n;
name = na;
score = s;
count++;
sum += s;
}
void show() {
cout << num << "\t" << name << "\t" << score << endl;
}
static void show_count_sum() {
cout << "count: " << count << "\tsum: " << sum << endl;
}
};
int student::count = 0;
float student::sum = 0;
int main() {
student s1(1, "Tom", 90.5);
student s2(2, "Jerry", 88.0);
student s3(3, "Tony", 95.0);
s1.show();
s2.show();
s3.show();
student::show_count_sum();
return 0;
}
```
程序的输出结果为:
```
1 Tom 90.5
2 Jerry 88
3 Tony 95
count: 3 sum: 273.5
```
程序的解释:
1. 定义了 `student` 类,数据成员包含 `num`(学号),`name`(姓名),`score`(成绩),`count`(人数),`sum`(总分)。
2. 定义了 `count`、`sum` 为静态数据成员,这样每个对象都可以共享这两个变量。
3. 定义了有参构造函数,用于初始化数据成员。在构造函数中增加了 `count` 和 `sum` 的值。
4. 定义了 `show()` 函数,用于输出学生的学号、姓名和成绩。
5. 定义了静态成员函数 `show_count_sum()`,用于输出人数和总分。
6. 在 `main()` 函数中定义了三个对象 `s1`、`s2`、`s3`,并调用了它们的 `show()` 函数输出学生的信息。
7. 最后调用了静态成员函数 `show_count_sum()`,输出了人数和总分。
相关推荐
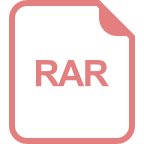
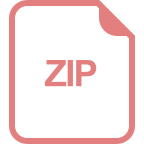
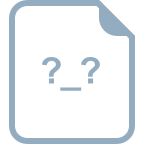














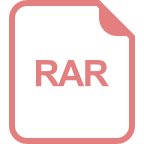