C# 用YOLO8训练自己的模型的代码 完整例程
时间: 2023-08-09 09:11:47 浏览: 111
好的,下面是一个使用C#编写的YOLOv3模型训练代码的例程,需要使用YOLOv3的darknet框架。请确保您已经安装了相关依赖和工具,并已经配置好相关环境变量。
```C#
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace YOLOv3
{
class Program
{
static void Main(string[] args)
{
string darknetPath = @"C:\darknet\"; // darknet框架路径
string cfgFile = @"C:\darknet\cfg\yolov3.cfg"; // 模型配置文件路径
string dataFile = @"C:\darknet\cfg\coco.data"; // 数据集配置文件路径
string weightsFile = @"C:\darknet\yolov3.weights"; // 预训练模型权重文件路径
string trainFile = @"C:\darknet\cfg\train.txt"; // 训练集文件路径
string validFile = @"C:\darknet\cfg\val.txt"; // 验证集文件路径
string backupPath = @"C:\darknet\backup\"; // 模型保存路径
// 训练模型命令
string trainCmd = $"{darknetPath}darknet.exe detector train {dataFile} {cfgFile} {weightsFile} -map -dont_show -mjpeg_port 8090 -gpus 0,1,2,3";
// 加载训练集和验证集
List<string> trainList = File.ReadAllLines(trainFile).ToList();
List<string> validList = File.ReadAllLines(validFile).ToList();
// 创建一个模型保存文件夹
if (!Directory.Exists(backupPath))
{
Directory.CreateDirectory(backupPath);
}
// 开始训练模型
var process = new System.Diagnostics.Process();
process.StartInfo.FileName = "cmd.exe";
process.StartInfo.CreateNoWindow = false;
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardInput = true;
process.StartInfo.RedirectStandardOutput = true;
process.StartInfo.RedirectStandardError = true;
process.Start();
process.StandardInput.WriteLine(trainCmd);
process.StandardInput.Flush();
process.StandardInput.Close();
string output = process.StandardOutput.ReadToEnd();
string error = process.StandardError.ReadToEnd();
process.WaitForExit();
process.Close();
// 输出训练结果
Console.WriteLine(output);
Console.WriteLine(error);
}
}
}
```
以上代码将模型训练所需的相关路径和命令进行了配置,并提供了一个简单的训练流程,以供参考。由于YOLOv3模型训练涉及到很多细节和参数调整,所以您可能需要根据自己的具体需求进行修改和调整。
阅读全文
相关推荐
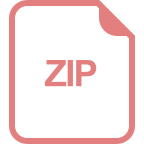
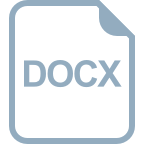

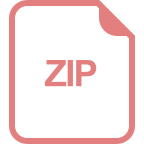

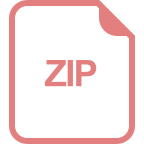
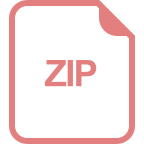
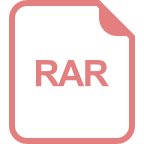
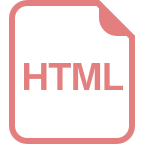
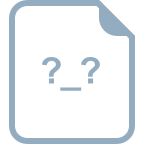
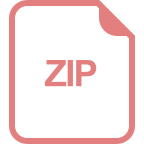
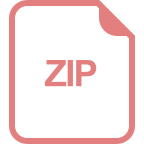
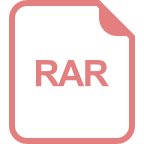
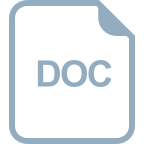
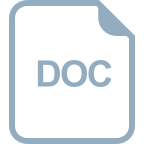
