请给我一个简单的“商品交易系统”前端的源代码
时间: 2023-08-06 14:09:15 浏览: 41
以下是一个简单的“商品交易系统”前端的HTML/CSS/JavaScript代码示例:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>商品交易系统</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<header>
<h1>商品交易系统</h1>
</header>
<main>
<section>
<h2>商品列表</h2>
<div id="goods-list"></div>
</section>
<section>
<h2>购物车</h2>
<div id="cart"></div>
<button id="checkout-btn">结算</button>
</section>
</main>
<footer>
<p>© 2021 商品交易系统</p>
</footer>
<script src="script.js"></script>
</body>
</html>
```
CSS代码:
```css
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: Arial, Helvetica, sans-serif;
}
header {
background-color: #333;
color: #fff;
padding: 20px;
}
header h1 {
margin: 0;
}
main {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
padding: 20px;
}
section {
flex: 0 1 45%;
margin-bottom: 20px;
}
section h2 {
margin-bottom: 10px;
}
#goods-list {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
grid-gap: 20px;
}
.goods-item {
border: 1px solid #ddd;
padding: 10px;
text-align: center;
}
.goods-item img {
max-width: 100%;
max-height: 200px;
margin-bottom: 10px;
}
.goods-item h3 {
margin-bottom: 10px;
}
.goods-item p {
margin-bottom: 10px;
}
#cart {
border: 1px solid #ddd;
padding: 10px;
text-align: center;
}
#cart table {
width: 100%;
}
#cart table th, #cart table td {
padding: 5px;
border: 1px solid #ddd;
}
#checkout-btn {
display: block;
margin: 20px auto;
padding: 10px 20px;
background-color: #333;
color: #fff;
border: none;
cursor: pointer;
}
footer {
background-color: #ddd;
padding: 20px;
text-align: center;
}
```
JavaScript代码:
```javascript
// 商品列表数据
const goodsData = [
{
id: 1,
name: '商品1',
description: '描述1',
price: 10.0,
quantity: 100,
image: 'https://via.placeholder.com/250x200.png?text=商品1',
},
{
id: 2,
name: '商品2',
description: '描述2',
price: 20.0,
quantity: 200,
image: 'https://via.placeholder.com/250x200.png?text=商品2',
},
{
id: 3,
name: '商品3',
description: '描述3',
price: 30.0,
quantity: 300,
image: 'https://via.placeholder.com/250x200.png?text=商品3',
},
];
// 购物车数据
let cartData = [];
// 获取商品列表DOM元素
const goodsListEl = document.getElementById('goods-list');
// 获取购物车DOM元素
const cartEl = document.getElementById('cart');
// 绘制商品列表
function drawGoodsList() {
goodsListEl.innerHTML = '';
goodsData.forEach(goods => {
const goodsItemEl = document.createElement('div');
goodsItemEl.classList.add('goods-item');
goodsItemEl.innerHTML = `
<img src="${goods.image}" alt="${goods.name}">
<h3>${goods.name}</h3>
<p>${goods.description}</p>
<p>价格:${goods.price}元</p>
<p>库存:${goods.quantity}</p>
<button onclick="addToCart(${goods.id})">加入购物车</button>
`;
goodsListEl.appendChild(goodsItemEl);
});
}
// 绘制购物车
function drawCart() {
cartEl.innerHTML = '';
const tableEl = document.createElement('table');
tableEl.innerHTML = `
<tr>
<th>商品名称</th>
<th>数量</th>
<th>单价</th>
<th>小计</th>
<th>操作</th>
</tr>
`;
let total = 0.0;
cartData.forEach(cartItem => {
const goods = goodsData.find(goods => goods.id === cartItem.id);
const subtotal = cartItem.quantity * goods.price;
total += subtotal;
const trEl = document.createElement('tr');
trEl.innerHTML = `
<td>${goods.name}</td>
<td>${cartItem.quantity}</td>
<td>${goods.price}元</td>
<td>${subtotal}元</td>
<td><button onclick="removeFromCart(${goods.id})">删除</button></td>
`;
tableEl.appendChild(trEl);
});
if (cartData.length === 0) {
tableEl.innerHTML += '<tr><td colspan="5">购物车为空</td></tr>';
}
else {
tableEl.innerHTML += `
<tr>
<td colspan="3">合计:</td>
<td>${total}元</td>
<td></td>
</tr>
`;
}
cartEl.appendChild(tableEl);
}
// 添加商品到购物车
function addToCart(goodsId) {
const cartItem = cartData.find(item => item.id === goodsId);
if (cartItem) {
cartItem.quantity += 1;
}
else {
cartData.push({ id: goodsId, quantity: 1 });
}
drawCart();
}
// 从购物车删除商品
function removeFromCart(goodsId) {
const cartItemIndex = cartData.findIndex(item => item.id === goodsId);
if (cartItemIndex >= 0) {
cartData.splice(cartItemIndex, 1);
}
drawCart();
}
// 结算
function checkout() {
alert('结算成功!');
cartData = [];
drawCart();
}
// 初始化
drawGoodsList();
drawCart();
document.getElementById('checkout-btn').addEventListener('click', checkout);
```
以上是一个简单的“商品交易系统”前端的HTML/CSS/JavaScript代码示例,您可以根据该示例进行编程实现。
相关推荐
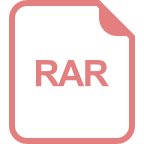














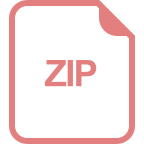