微信小程序支付功能 C#
时间: 2023-07-13 19:29:48 浏览: 189
微信小程序支付功能的实现可以分为以下几个步骤:
1. 获取用户授权:在小程序中调用 wx.login 接口获取 code,然后使用 code 调用 wx.getUserInfo 接口获取用户信息,最后将用户信息传递给后端服务器。
2. 后端服务器获取用户 openid:后端服务器使用微信开放平台提供的接口,根据用户授权时获取的 code,获取用户 openid。
3. 生成支付订单:后端服务器使用用户 openid 和商品信息生成支付订单,将订单信息返回给小程序。
4. 小程序调起支付:小程序使用微信提供的 wx.requestPayment 接口,将支付订单信息传递给微信支付,微信支付会生成一个支付结果。
5. 支付结果处理:微信支付会将支付结果返回给小程序,小程序将支付结果传递给后端服务器,后端服务器根据支付结果更新订单状态。
在 C# 中实现微信小程序支付功能,需要使用微信提供的支付 API,同时需要使用 C# 的网络编程和加密解密技术。具体实现步骤可以参考微信支付开发文档和 C# 网络编程和加密解密相关的文档。
相关问题
c# 对接通联的微信小程序支付代码
以下是一个C#对接通联微信小程序支付的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.IO;
using System.Xml;
namespace WeChatPay
{
class Program
{
static void Main(string[] args)
{
string strAppid = "你的小程序的appid";
string strMchId = "你的商户号";
string strKey = "你的API密钥";
string strNonce = Guid.NewGuid().ToString().Replace("-", "");
string strBody = "商品描述";
string strOutTradeNo = "商户订单号";
string strTotalFee = "订单总金额,单位为分";
string strSpbillCreateIp = "调用微信支付API的机器的IP地址";
string strNotifyUrl = "接收微信支付异步通知回调地址";
string strTradeType = "JSAPI";
string strOpenid = "用户在商户appid下的唯一标识";
string strSign = "";
//生成签名
SortedDictionary<string, string> dic = new SortedDictionary<string, string>();
dic.Add("appid", strAppid);
dic.Add("mch_id", strMchId);
dic.Add("nonce_str", strNonce);
dic.Add("body", strBody);
dic.Add("out_trade_no", strOutTradeNo);
dic.Add("total_fee", strTotalFee);
dic.Add("spbill_create_ip", strSpbillCreateIp);
dic.Add("notify_url", strNotifyUrl);
dic.Add("trade_type", strTradeType);
dic.Add("openid", strOpenid);
string strSignTemp = "";
foreach (KeyValuePair<string, string> kvp in dic)
{
if (!string.IsNullOrEmpty(kvp.Value))
{
strSignTemp += kvp.Key + "=" + kvp.Value + "&";
}
}
strSignTemp += "key=" + strKey;
strSign = System.Web.Security.FormsAuthentication.HashPasswordForStoringInConfigFile(strSignTemp, "MD5").ToUpper();
//生成XML请求参数
string strXml = "<xml>"
+ "<appid>" + strAppid + "</appid>"
+ "<mch_id>" + strMchId + "</mch_id>"
+ "<nonce_str>" + strNonce + "</nonce_str>"
+ "<body>" + strBody + "</body>"
+ "<out_trade_no>" + strOutTradeNo + "</out_trade_no>"
+ "<total_fee>" + strTotalFee + "</total_fee>"
+ "<spbill_create_ip>" + strSpbillCreateIp + "</spbill_create_ip>"
+ "<notify_url>" + strNotifyUrl + "</notify_url>"
+ "<trade_type>" + strTradeType + "</trade_type>"
+ "<openid>" + strOpenid + "</openid>"
+ "<sign>" + strSign + "</sign>"
+ "</xml>";
//向微信支付接口发送请求
string url = "https://api.mch.weixin.qq.com/pay/unifiedorder";
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
byte[] data = Encoding.UTF8.GetBytes(strXml);
request.ContentLength = data.Length;
Stream stream = request.GetRequestStream();
stream.Write(data, 0, data.Length);
stream.Close();
//获取微信支付接口返回的数据
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
StreamReader reader = new StreamReader(response.GetResponseStream(), Encoding.UTF8);
string strResult = reader.ReadToEnd();
XmlDocument xmlResult = new XmlDocument();
xmlResult.LoadXml(strResult);
//解析返回数据,获取prepay_id
XmlNode xmlNode = xmlResult.SelectSingleNode("xml/prepay_id");
string strPrepayId = xmlNode.InnerText;
//生成小程序支付需要的参数
SortedDictionary<string, string> dicPay = new SortedDictionary<string, string>();
dicPay.Add("appId", strAppid);
dicPay.Add("nonceStr", strNonce);
dicPay.Add("package", "prepay_id=" + strPrepayId);
dicPay.Add("signType", "MD5");
dicPay.Add("timeStamp", Convert.ToInt64(DateTime.Now.Subtract(new DateTime(1970, 1, 1)).TotalSeconds).ToString());
string strSignTempPay = "";
foreach (KeyValuePair<string, string> kvp in dicPay)
{
if (!string.IsNullOrEmpty(kvp.Value))
{
strSignTempPay += kvp.Key + "=" + kvp.Value + "&";
}
}
strSignTempPay += "key=" + strKey;
string strPaySign = System.Web.Security.FormsAuthentication.HashPasswordForStoringInConfigFile(strSignTempPay, "MD5").ToUpper();
//返回小程序支付所需参数
Console.WriteLine("appId:" + strAppid);
Console.WriteLine("nonceStr:" + strNonce);
Console.WriteLine("package:" + "prepay_id=" + strPrepayId);
Console.WriteLine("signType:MD5");
Console.WriteLine("timeStamp:" + dicPay["timeStamp"]);
Console.WriteLine("paySign:" + strPaySign);
}
}
}
```
请注意替换代码中的变量值为您自己的实际值。此代码仅供参考,具体实现方式可能因为不同的商户号、API密钥等因素而有所不同。
c# 微信小程序 微信支付 源代码
对于"C"这个字母,它在英语中代表着很多不同的含义。首先,它是英语字母表中的第三个字母,也是许多单词的首字母,比如cat(猫)、car(汽车)和coffee(咖啡)。在计算机科学领域,"C"也可以代表一种编程语言,它是一种广泛使用的通用程序设计语言,被广泛用于系统软件、应用程序和嵌入式系统的开发。此外,C还代表着一些数学和物理学中的常数,比如圆周率π的英文首字母就是"C"。在音乐领域,C也是一个音名,代表着音阶中的第一音。此外,在罗马数字中,"C"代表着100这个数值。总的来说,"C"是一个非常多功能和多义的字母,它在不同的领域都具有不同的意义和用途。
阅读全文
相关推荐
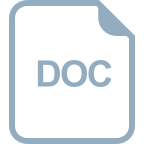
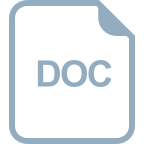
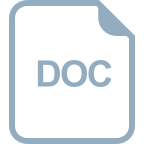
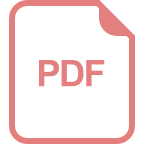
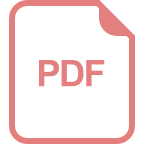
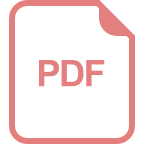
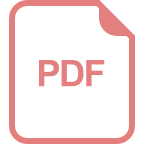
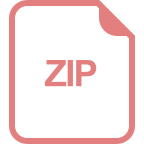
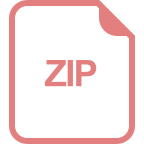
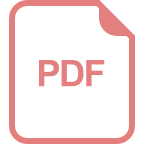
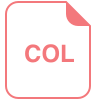
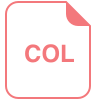
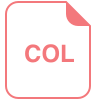
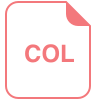

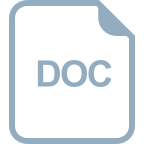
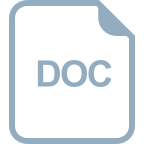