如何在生成的词云图片上点击词语并显示次数
时间: 2024-02-27 09:55:47 浏览: 28
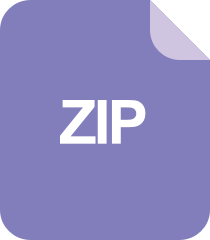
基于Python实现的词云生成代码,从txt文件中读取词语,生成指定图片的词云图
要实现在词云图片上点击词语并显示次数,您可以使用`matplotlib`的事件处理功能和`wordcloud`的`process_text`方法。具体实现步骤如下:
1. 首先导入需要的包:
```
import numpy as np
from PIL import Image
from wordcloud import WordCloud, STOPWORDS, ImageColorGenerator
import matplotlib.pyplot as plt
```
2. 加载文本数据并创建一个`WordCloud`对象:
```
text = open('your_text_file.txt', 'r').read()
# 创建WordCloud对象
wc = WordCloud(background_color="white", max_words=2000, contour_width=3, contour_color='steelblue')
wc.generate(text)
```
3. 创建一个`matplotlib`的图表并将词云对象绘制在上面:
```
plt.figure(figsize=(10, 10))
plt.imshow(wc, interpolation='bilinear')
plt.axis("off")
plt.show()
```
4. 创建一个`process_text`函数,用于处理词语的出现次数:
```
def process_text(word_string):
words = word_string.split()
d = {}
for word in words:
if word in d:
d[word] += 1
else:
d[word] = 1
return d
```
5. 创建一个`onclick`函数,用于在点击词语时显示它的出现次数:
```
def onclick(event):
x, y = event.x, event.y
print(x, y)
word = wc.get_array()[y, x]
word_string = ' '.join(wc.words_)
d = process_text(word_string)
if word in d:
print(f"\"{word}\" appears {d[word]} times.")
else:
print(f"\"{word}\" does not appear in the text.")
```
6. 使用`matplotlib`的`connect`方法将`onclick`函数连接到图表的`button_press_event`事件:
```
plt.gcf().canvas.mpl_connect('button_press_event', onclick)
```
完成上述步骤后,您可以点击词云图片上的任何一个词语,控制台将输出该词语在文本中出现的次数。
阅读全文
相关推荐
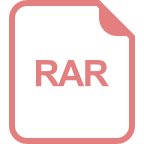
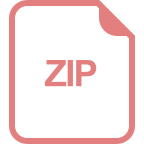
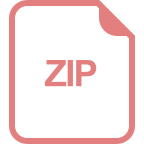
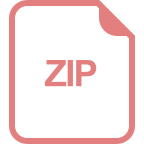
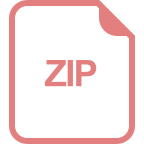












