使用python产生车联网环境下车辆行驶数据,给定车辆总数1000,产生一定时间内的时间序列,产生对应时间序列的速度,计算每辆车辆位置,绘制所有车辆的速度-时间-车辆编号三维可视化图形,绘制所有车辆的位置-时间-车辆编号三维可视化图形
时间: 2024-03-25 12:35:19 浏览: 28
好的,让我来回答你的问题。
对于你的问题,需要用到Python的模拟数据功能来产生车辆行驶数据。可以使用Python的numpy和pandas库来生成随机的车辆速度数据,然后使用时间序列函数来生成时间戳数据。具体实现如下:
```python
import numpy as np
import pandas as pd
# 生成1000辆车的速度数据
car_speeds = np.random.normal(loc=60, scale=10, size=1000)
# 生成时间戳数据,每1秒钟一个时间戳,共10小时
timestamp = pd.date_range(start='2022-01-01', end='2022-01-01 10:00:00', freq='S')
# 生成每辆车在每个时间戳下的位置
# 假设所有车辆都从0位置出发,匀速行驶
car_positions = np.zeros((1000, len(timestamp)))
for i in range(len(car_speeds)):
car_positions[i,:] = np.arange(0, len(timestamp)) * car_speeds[i] / 3600
# 用matplotlib库绘制所有车辆的速度-时间-车辆编号三维可视化图形
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
for i in range(len(car_speeds)):
ax.plot(timestamp, [i]*len(timestamp), car_speeds[i], color='blue')
ax.set_xlabel('Time')
ax.set_ylabel('Car ID')
ax.set_zlabel('Speed')
plt.show()
# 用matplotlib库绘制所有车辆的位置-时间-车辆编号三维可视化图形
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
for i in range(len(car_speeds)):
ax.plot(timestamp, [i]*len(timestamp), car_positions[i,:], color='red')
ax.set_xlabel('Time')
ax.set_ylabel('Car ID')
ax.set_zlabel('Position')
plt.show()
```
这样就可以生成车辆行驶数据,并绘制可视化图形了。
阅读全文
相关推荐
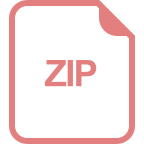
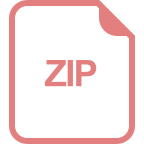


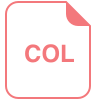
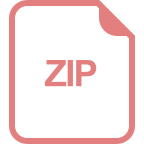
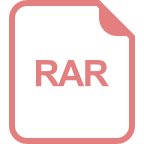
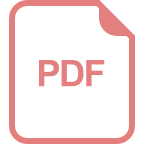
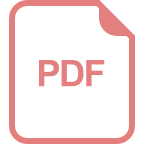
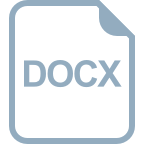
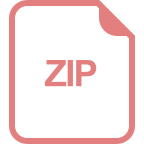
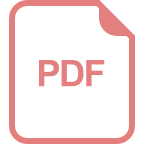
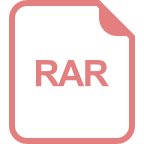
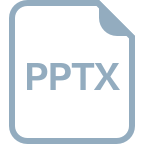
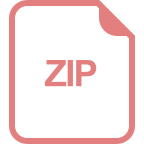
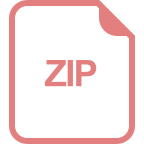