java合并不同目录下的txt文件
时间: 2023-10-27 20:20:59 浏览: 77
可以使用Java的IO操作,遍历不同目录下的txt文件,读取文件内容并将其合并到一个文件中。以下是一个简单的示例代码:
```java
import java.io.*;
public class MergeTxtFiles {
public static void main(String[] args) {
// 定义要合并的目录路径
String dirPath = "D:/txtfiles/";
// 定义要合并的文件名前缀
String fileNamePrefix = "file";
// 定义要合并的文件数量
int fileCount = 3;
// 定义合并后的文件名
String mergedFileName = "merged.txt";
// 创建合并文件
File mergedFile = new File(dirPath + mergedFileName);
try {
mergedFile.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
// 遍历目录下的文件并将其合并到合并文件中
BufferedWriter writer = null;
try {
writer = new BufferedWriter(new FileWriter(mergedFile, true));
for (int i = 1; i <= fileCount; i++) {
File file = new File(dirPath + fileNamePrefix + i + ".txt");
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
writer.write(line + "\n");
}
reader.close();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
在上面的示例代码中,我们定义了要合并的目录路径、文件名前缀、文件数量以及合并后的文件名,并创建了合并文件。然后,我们遍历目录下的文件,将其内容逐行写入合并文件中。最后关闭写入流即可完成文件合并。
阅读全文
相关推荐






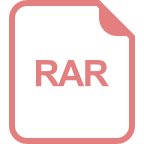








