用vb.net写一段代码,要求可以根据点坐标和坐标对应值进行插值做出相应的彩色云图,并画到picturebox里
时间: 2024-03-08 07:46:47 浏览: 21
以下是一个简单的用 VB.NET 实现插值并绘制彩色云图的示例代码,使用了 bilinear 插值算法:
```
' 定义插值函数
Function Interpolate(x As Double, y As Double, values As Double(,), xvals As Double(), yvals As Double()) As Double
Dim xsize As Integer = xvals.Length
Dim ysize As Integer = yvals.Length
Dim xIndex As Integer = Array.BinarySearch(xvals, x)
Dim yIndex As Integer = Array.BinarySearch(yvals, y)
If xIndex < 0 Then
xIndex = (~xIndex) - 1
End If
If yIndex < 0 Then
yIndex = (~yIndex) - 1
End If
If xIndex < 0 OrElse xIndex >= xsize - 1 OrElse yIndex < 0 OrElse yIndex >= ysize - 1 Then
Return Double.NaN
End If
Dim x1 As Double = xvals(xIndex)
Dim x2 As Double = xvals(xIndex + 1)
Dim y1 As Double = yvals(yIndex)
Dim y2 As Double = yvals(yIndex + 1)
Dim f11 As Double = values(xIndex, yIndex)
Dim f12 As Double = values(xIndex, yIndex + 1)
Dim f21 As Double = values(xIndex + 1, yIndex)
Dim f22 As Double = values(xIndex + 1, yIndex + 1)
Dim dx As Double = (x - x1) / (x2 - x1)
Dim dy As Double = (y - y1) / (y2 - y1)
Dim f1 As Double = f11 * (1 - dx) + f21 * dx
Dim f2 As Double = f12 * (1 - dx) + f22 * dx
Return f1 * (1 - dy) + f2 * dy
End Function
' 生成彩色云图
Sub GenerateCloudMap(values As Double(,), xvals As Double(), yvals As Double(), pictureBox As PictureBox)
Dim width As Integer = pictureBox.Width
Dim height As Integer = pictureBox.Height
Dim bitmap As New Bitmap(width, height)
' 计算最小值和最大值
Dim minVal As Double = Double.MaxValue
Dim maxVal As Double = Double.MinValue
For i = 0 To xvals.Length - 1
For j = 0 To yvals.Length - 1
Dim val = values(i, j)
If Not Double.IsNaN(val) Then
If val < minVal Then
minVal = val
End If
If val > maxVal Then
maxVal = val
End If
End If
Next
Next
' 绘制彩色云图
For i = 0 To width - 1
For j = 0 To height - 1
Dim x As Double = xvals(0) + (i / width) * (xvals(xvals.Length - 1) - xvals(0))
Dim y As Double = yvals(0) + (j / height) * (yvals(yvals.Length - 1) - yvals(0))
Dim val As Double = Interpolate(x, y, values, xvals, yvals)
If Not Double.IsNaN(val) Then
Dim r As Integer = 0
Dim g As Integer = 0
Dim b As Integer = 0
' 根据数值计算颜色
If maxVal > minVal Then
Dim t As Double = (val - minVal) / (maxVal - minVal)
If t < 0.25 Then
r = 0
g = CInt(t * 1020)
b = 255
ElseIf t < 0.5 Then
r = 0
g = 255
b = CInt((0.5 - t) * 1020)
ElseIf t < 0.75 Then
r = CInt((t - 0.5) * 1020)
g = 255
b = 0
Else
r = 255
g = CInt((1 - t) * 1020)
b = 0
End If
End If
bitmap.SetPixel(i, j, Color.FromArgb(r, g, b))
End If
Next
Next
' 显示图片
pictureBox.Image = bitmap
End Sub
```
使用方法:
1. 将上述代码复制到你的 VB.NET 项目中。
2. 准备好点坐标和坐标对应值,保存成二维数组 values,x 坐标数组 xvals 和 y 坐标数组 yvals。
3. 调用 GenerateCloudMap 函数,传入以上三个参数和 PictureBox 控件对象,即可生成彩色云图并显示在 PictureBox 中。
示例代码中使用 bilinear 插值算法,如果需要使用其他插值算法可以自行实现。另外,颜色映射是根据数值线性映射到颜色空间的,可以根据需要自行修改。
相关推荐
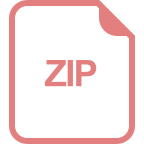














