鲸鱼算法python代码
时间: 2023-08-18 19:06:08 浏览: 200
这是一个简单的鲸鱼算法的Python代码示例:
```python
import random
import math
# 鲸鱼算法实现类
class WhaleOptimizationAlgorithm:
def __init__(self, num_of_search_agents, max_iter, dim, obj_func):
self.num_of_search_agents = num_of_search_agents
self.max_iter = max_iter
self.dim = dim
self.obj_func = obj_func
# 初始化种群
def init_population(self):
return [[random.uniform(-10, 10) for j in range(self.dim)] for i in range(self.num_of_search_agents)]
# 计算适应度
def fitness(self, x):
return self.obj_func(x)
# 鲸鱼算法实现函数
def optimize(self):
# 初始化种群
X = self.init_population()
# 最优解
best_solution_fitness = float("inf")
best_solution_idx = -1
best_solution = []
# 迭代
for t in range(self.max_iter):
a = 2 - t * ((2) / self.max_iter)
# 对每个鲸鱼进行迭代
for i in range(self.num_of_search_agents):
r1 = random.random()
r2 = random.random()
A = 2 * a * r1 - a
C = 2 * r2
# 寻找当前最优解
if random.random() >= 0.5:
D = abs(C * best_solution - X[i])
X[i] = best_solution - A * D
else:
D1 = abs(best_solution - X[i])
D2 = abs(self.best_solution - X[i])
X[i] = D1 * math.exp(b * C) * math.cos(2 * math.pi * C) + best_solution
# 边界处理
for j in range(self.dim):
X[i][j] = max(min(X[i][j], 10), -10)
# 计算适应度
fitness = self.fitness(X[i])
# 更新最优解
if fitness < best_solution_fitness:
best_solution_fitness = fitness
best_solution_idx = i
best_solution = X[i]
return (best_solution, best_solution_fitness)
```
其中,`num_of_search_agents` 表示搜索代理的数量,`max_iter` 表示最大迭代次数,`dim` 表示搜索空间的维度,`obj_func` 是目标函数。在 `optimize()` 函数中,我们首先初始化种群,然后进行迭代,对每个鲸鱼进行操作,更新最优解。最后返回最优解及其适应度。
阅读全文
相关推荐
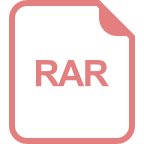




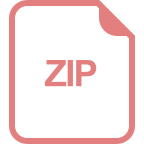
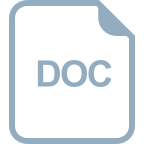






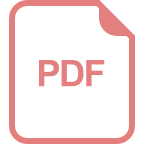