给我看看Python gnupg使用指南
时间: 2023-11-05 09:22:05 浏览: 49
以下是 Python gnupg 使用指南:
1. 安装 gnupg 模块
通过 pip 安装 gnupg 模块:
```
pip install python-gnupg
```
2. 导入 gnupg 模块
```python
import gnupg
```
3. 创建 GPG 对象
```python
gpg = gnupg.GPG()
```
创建 GPG 对象时,可以使用 `gnupghome` 参数指定 GnuPG 的主目录,例如:
```python
gpg = gnupg.GPG(gnupghome='/path/to/gnupg/home')
```
4. 导入公钥
```python
with open('public_key.asc', 'rb') as f:
public_key = f.read()
import_result = gpg.import_keys(public_key)
print(import_result.results)
```
在上述示例中,我们使用 `open()` 函数打开公钥文件,读取公钥内容,并使用 `gpg.import_keys()` 方法将公钥导入到 GPG 对象中。`import_result` 变量是一个 `ImportResult` 对象,包含了导入结果的详细信息。
5. 加密数据
```python
data = b'Hello, world!'
encrypted_data = gpg.encrypt(data, import_result.fingerprints[0], always_trust=True)
print(encrypted_data.data)
```
在上述示例中,我们使用 `gpg.encrypt()` 方法对数据进行加密,其中 `data` 是要加密的数据,`import_result.fingerprints[0]` 是接收方的指纹(即密钥 ID),`always_trust=True` 表示信任接收方的公钥。
加密结果保存在 `encrypted_data.data` 中,可以将其发送给接收方。
6. 解密数据
```python
decrypted_data = gpg.decrypt(encrypted_data.data, passphrase='your_passphrase')
print(decrypted_data.data)
```
在上述示例中,我们使用 `gpg.decrypt()` 方法对加密数据进行解密,其中 `encrypted_data.data` 是要解密的数据,`passphrase='your_passphrase'` 表示私钥密码。
解密结果保存在 `decrypted_data.data` 中,可以对其进行处理。
7. 签名数据
```python
signed_data = gpg.sign(data, keyid=import_result.fingerprints[0], passphrase='your_passphrase')
print(signed_data.data)
```
在上述示例中,我们使用 `gpg.sign()` 方法对数据进行签名,其中 `data` 是要签名的数据,`keyid=import_result.fingerprints[0]` 表示使用指定的密钥 ID 进行签名,`passphrase='your_passphrase'` 表示私钥密码。
签名结果保存在 `signed_data.data` 中,可以将其发送给接收方。
8. 验证签名
```python
verification_result = gpg.verify(signed_data.data)
print(verification_result)
```
在上述示例中,我们使用 `gpg.verify()` 方法对签名进行验证,其中 `signed_data.data` 是要验证的签名数据。
验证结果保存在 `verification_result` 变量中,是一个 `VerifyResult` 对象,包含了验证结果的详细信息。
9. 生成密钥对
```python
input_data = gpg.gen_key_input(name_email='your_name <your_email>', passphrase='your_passphrase')
key = gpg.gen_key(input_data)
ascii_armored_public_key = gpg.export_keys(key.fingerprint)
ascii_armored_private_key = gpg.export_keys(key.fingerprint, True, passphrase='your_passphrase')
print(ascii_armored_public_key)
print(ascii_armored_private_key)
```
在上述示例中,我们使用 `gpg.gen_key_input()` 方法生成密钥对的输入数据,然后使用 `gpg.gen_key()` 方法生成密钥对。最后,我们使用 `gpg.export_keys()` 方法导出公钥和私钥,其中 `True` 表示导出私钥。
需要注意的是,生成密钥对时需要提供一些必要的信息,例如名称、电子邮件地址和密码等。另外,导出私钥时需要提供私钥密码。
以上就是使用 Python gnupg 模块进行加密、解密、签名和验证等操作的基本步骤。
相关推荐
















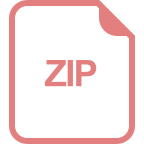
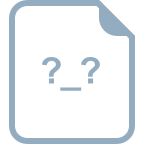