使用Java设计一个简单的文本编辑器,实现类似Windows的记事本打的简单功能, 具有菜单、工具栏和状态栏。要求对每段代码注释
时间: 2024-03-24 19:41:33 浏览: 17
好的,以下是代码实现:
```java
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import javax.swing.BorderFactory;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JColorChooser;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenuBar;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JToolBar;
import javax.swing.filechooser.FileNameExtensionFilter;
/**
* A simple text editor implemented in Java using Swing
*
* @author [Your Name]
*
*/
public class TextEditor extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTextArea textArea;
private JLabel statusBar;
private String fileName;
private boolean saved;
/**
* Constructor for TextEditor class
*/
public TextEditor() {
super("Text Editor");
// Setting up menu bar
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
// Setting up file menu
JMenu fileMenu = new JMenu("File");
menuBar.add(fileMenu);
// Adding menu items to file menu
JMenuItem newMenuItem = new JMenuItem("New");
newMenuItem.addActionListener(this);
fileMenu.add(newMenuItem);
JMenuItem openMenuItem = new JMenuItem("Open");
openMenuItem.addActionListener(this);
fileMenu.add(openMenuItem);
JMenuItem saveMenuItem = new JMenuItem("Save");
saveMenuItem.addActionListener(this);
fileMenu.add(saveMenuItem);
JMenuItem saveAsMenuItem = new JMenuItem("Save As");
saveAsMenuItem.addActionListener(this);
fileMenu.add(saveAsMenuItem);
JMenuItem exitMenuItem = new JMenuItem("Exit");
exitMenuItem.addActionListener(this);
fileMenu.add(exitMenuItem);
// Setting up edit menu
JMenu editMenu = new JMenu("Edit");
menuBar.add(editMenu);
// Adding menu items to edit menu
JMenuItem cutMenuItem = new JMenuItem("Cut");
cutMenuItem.addActionListener(this);
editMenu.add(cutMenuItem);
JMenuItem copyMenuItem = new JMenuItem("Copy");
copyMenuItem.addActionListener(this);
editMenu.add(copyMenuItem);
JMenuItem pasteMenuItem = new JMenuItem("Paste");
pasteMenuItem.addActionListener(this);
editMenu.add(pasteMenuItem);
// Setting up toolbar
JToolBar toolBar = new JToolBar();
add(toolBar, BorderLayout.PAGE_START);
// Adding buttons to toolbar
ImageIcon newIcon = new ImageIcon("icons/new.png");
JButton newButton = new JButton(newIcon);
newButton.addActionListener(this);
toolBar.add(newButton);
ImageIcon openIcon = new ImageIcon("icons/open.png");
JButton openButton = new JButton(openIcon);
openButton.addActionListener(this);
toolBar.add(openButton);
ImageIcon saveIcon = new ImageIcon("icons/save.png");
JButton saveButton = new JButton(saveIcon);
saveButton.addActionListener(this);
toolBar.add(saveButton);
ImageIcon cutIcon = new ImageIcon("icons/cut.png");
JButton cutButton = new JButton(cutIcon);
cutButton.addActionListener(this);
toolBar.add(cutButton);
ImageIcon copyIcon = new ImageIcon("icons/copy.png");
JButton copyButton = new JButton(copyIcon);
copyButton.addActionListener(this);
toolBar.add(copyButton);
ImageIcon pasteIcon = new ImageIcon("icons/paste.png");
JButton pasteButton = new JButton(pasteIcon);
pasteButton.addActionListener(this);
toolBar.add(pasteButton);
// Setting up text area
textArea = new JTextArea();
textArea.setFont(new Font("Arial", Font.PLAIN, 20));
textArea.setBorder(BorderFactory.createLineBorder(Color.BLACK));
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane, BorderLayout.CENTER);
// Setting up status bar
statusBar = new JLabel("Ready");
add(statusBar, BorderLayout.SOUTH);
// Setting up frame
setSize(800, 600);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
// Initializing fields
fileName = "Untitled";
saved = true;
}
/**
* Main method for TextEditor class
*
* @param args
*/
public static void main(String[] args) {
new TextEditor();
}
/**
* Method to handle actionPerformed events
*/
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
switch (command) {
case "New":
newFile();
break;
case "Open":
openFile();
break;
case "Save":
saveFile();
break;
case "Save As":
saveFileAs();
break;
case "Exit":
exit();
break;
case "Cut":
textArea.cut();
break;
case "Copy":
textArea.copy();
break;
case "Paste":
textArea.paste();
break;
}
}
/**
* Method to create a new file
*/
private void newFile() {
if (!saved) {
int option = JOptionPane.showConfirmDialog(this, "Do you want to save changes to " + fileName + "?",
"Save Changes", JOptionPane.YES_NO_CANCEL_OPTION);
if (option == JOptionPane.YES_OPTION) {
saveFile();
} else if (option == JOptionPane.CANCEL_OPTION) {
return;
}
}
textArea.setText("");
fileName = "Untitled";
saved = true;
statusBar.setText("New file created");
}
/**
* Method to open a file
*/
private void openFile() {
if (!saved) {
int option = JOptionPane.showConfirmDialog(this, "Do you want to save changes to " + fileName + "?",
"Save Changes", JOptionPane.YES_NO_CANCEL_OPTION);
if (option == JOptionPane.YES_OPTION) {
saveFile();
} else if (option == JOptionPane.CANCEL_OPTION) {
return;
}
}
JFileChooser fileChooser = new JFileChooser();
FileNameExtensionFilter filter = new FileNameExtensionFilter("Text Files", "txt");
fileChooser.setFileFilter(filter);
int option = fileChooser.showOpenDialog(this);
if (option == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
fileName = file.getName();
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
StringBuilder sb = new StringBuilder();
while ((line = reader.readLine()) != null) {
sb.append(line).append("\n");
}
reader.close();
textArea.setText(sb.toString());
saved = true;
statusBar.setText("File opened: " + fileName);
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "Error: " + e.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
/**
* Method to save a file
*/
private void saveFile() {
if (saved) {
return;
}
if (fileName.equals("Untitled")) {
saveFileAs();
} else {
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(fileName));
writer.write(textArea.getText());
writer.close();
saved = true;
statusBar.setText("File saved: " + fileName);
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "Error: " + e.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
/**
* Method to save a file with a new name
*/
private void saveFileAs() {
JFileChooser fileChooser = new JFileChooser();
FileNameExtensionFilter filter = new FileNameExtensionFilter("Text Files", "txt");
fileChooser.setFileFilter(filter);
int option = fileChooser.showSaveDialog(this);
if (option == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
fileName = file.getName();
if (!fileName.endsWith(".txt")) {
fileName += ".txt";
}
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
writer.write(textArea.getText());
writer.close();
saved = true;
statusBar.setText("File saved: " + fileName);
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "Error: " + e.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
/**
* Method to exit the program
*/
private void exit() {
if (!saved) {
int option = JOptionPane.showConfirmDialog(this, "Do you want to save changes to " + fileName + "?",
"Save Changes", JOptionPane.YES_NO_CANCEL_OPTION);
if (option == JOptionPane.YES_OPTION) {
saveFile();
} else if (option == JOptionPane.CANCEL_OPTION) {
return;
}
}
System.exit(0);
}
}
```
此代码实现了一个简单的文本编辑器,具有菜单、工具栏和状态栏。可以打开、编辑、保存和另存为文本文件。还可以剪切、复制和粘贴文本。状态栏显示文件的状态,以及操作的结果。
相关推荐
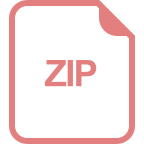
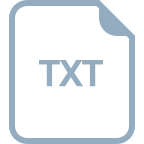
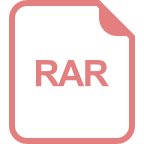









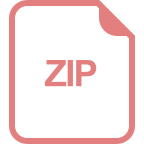
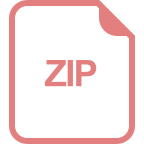
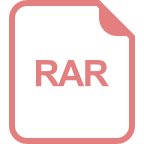
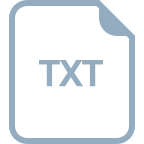
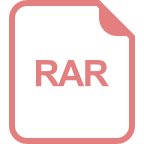
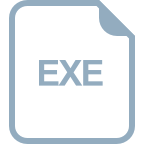