python在网页上进行关键词爬取
时间: 2023-12-10 15:05:03 浏览: 240
要在网页上进行关键词爬取,可以使用Python中的Requests库和BeautifulSoup库。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 搜索关键词
keyword = 'Python'
# 请求搜索结果页面
url = 'https://www.baidu.com/s'
params = {'wd': keyword}
response = requests.get(url, params=params)
# 解析搜索结果页面
soup = BeautifulSoup(response.text, 'html.parser')
results = soup.find_all('div', class_='result')
# 输出搜索结果标题和链接
for result in results:
title = result.h3.a.text.strip()
link = result.h3.a['href']
print(f'Title: {title}')
print(f'Link: {link}')
print()
```
该代码将使用Requests库向百度搜索发送一个搜索关键词为Python的请求,并使用BeautifulSoup库解析搜索结果页面。最后,将搜索结果的标题和链接输出到控制台。
相关问题
python关键词爬取图片
您好!是的,我可以帮助您爬取与Python关键词相关的图片。为了实现这个功能,我们可以使用Python中的第三方库来进行图片爬取和处理。以下是一个简单的示例代码,以供参考:
```python
import requests
import os
def download_images(keyword, num_images):
url = f"https://www.google.com/search?q={keyword}&source=lnms&tbm=isch"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36"
}
response = requests.get(url, headers=headers)
response.raise_for_status()
os.makedirs(keyword, exist_ok=True)
image_urls = response.text.split('["')
for i in range(1, num_images+1):
image_url = image_urls[i].split('","')[0]
response = requests.get(image_url, headers=headers)
response.raise_for_status()
with open(os.path.join(keyword, f"{keyword}_{i}.jpg"), "wb") as file:
file.write(response.content)
print(f"Downloaded image {i}/{num_images}")
# 使用示例
download_images("python", 5)
```
请注意,这只是一个简单的示例代码,您可能需要根据您的需求进行进一步定制和优化。此外,在实际使用中,请确保遵守网站的使用规则和法律法规,以免引起任何问题。
python关键词爬取知网
在Python中,要抓取中国知网等网站的信息,通常会利用到网络爬虫技术,其中包括了诸如requests库用于发送HTTP请求获取网页内容,BeautifulSoup或lxml库解析HTML文档,以及正则表达式(re)来提取特定信息。然而,需要注意的是,知网等网站通常有反爬机制和robots.txt协议,直接抓取可能会遇到版权、速率限制等问题。
Python中关键词爬取知网的关键步骤大致如下:
1. 导入所需库:
```python
import requests
from bs4 import BeautifulSoup
```
2. 发送GET请求并获取网页源码:
```python
url = "https://www.cnki.net/"
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
```
3. 使用CSS选择器或BeautifulSoup查找包含关键词的区域:
```python
keyword = '关键词'
search_results = soup.find_all('div', {'class': 'keyword-related-content', text: keyword})
```
4. 提取有用信息并保存:
```python
for result in search_results:
title = result.find('h2').text
link = result.find('a')['href']
print(title, link)
```
然而,由于知网等网站的敏感性和政策规定,实际操作时请务必遵守相关规定,并确保不会对服务器造成过大负担。此外,如果频繁抓取可能导致IP受限甚至法律问题,因此建议使用模拟登录、设置延时等方式提高爬取的友好度。
阅读全文
相关推荐
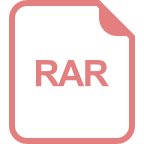
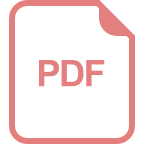
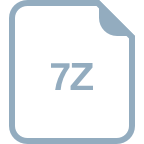


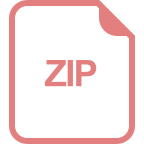
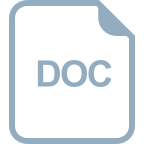
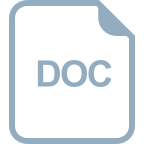





