用c++写一个栈,要求实现判断栈是否为空、出栈入栈、遍历栈、返回元素、销毁栈等功能,实现多态、运算符重载和文件操作,并改为模板
时间: 2023-07-10 22:34:36 浏览: 63
好的,我来为你编写一个C++模板类实现栈的功能,包括判断栈是否为空、出栈入栈、遍历栈、返回元素、销毁栈等功能,同时支持多态、运算符重载和文件操作。
```c++
#include <iostream>
#include <fstream>
using namespace std;
template <typename T>
class Stack {
private:
T* stack_ptr;
int size;
int top_index;
public:
Stack(int s) {
size = s;
stack_ptr = new T[size];
top_index = -1;
}
Stack() {
size = 10;
stack_ptr = new T[size];
top_index = -1;
}
void push(T element) {
if (isFull()) {
cout << "Stack is full. Cannot push element." << endl;
return;
}
stack_ptr[++top_index] = element;
}
T pop() {
if (isEmpty()) {
cout << "Stack is empty. Cannot pop element." << endl;
return 0;
}
return stack_ptr[top_index--];
}
T peek() {
if (isEmpty()) {
cout << "Stack is empty. Cannot peek element." << endl;
return 0;
}
return stack_ptr[top_index];
}
bool isEmpty() {
return (top_index == -1);
}
bool isFull() {
return (top_index == size - 1);
}
void print() {
if (isEmpty()) {
cout << "Stack is empty." << endl;
return;
}
cout << "Stack elements: ";
for (int i = 0; i <= top_index; i++) {
cout << stack_ptr[i] << " ";
}
cout << endl;
}
T& operator[](int index) {
if (index < 0 || index > top_index) {
cout << "Index out of range. Cannot access element." << endl;
}
return stack_ptr[index];
}
void operator=(Stack<T>& s) {
delete[] stack_ptr;
size = s.size;
stack_ptr = new T[size];
top_index = s.top_index;
for (int i = 0; i <= top_index; i++) {
stack_ptr[i] = s.stack_ptr[i];
}
}
void saveToFile(string filename) {
ofstream file(filename);
file << size << " " << top_index << endl;
for (int i = 0; i <= top_index; i++) {
file << stack_ptr[i] << " ";
}
file.close();
}
void readFromFile(string filename) {
ifstream file(filename);
int s, t;
file >> s >> t;
delete[] stack_ptr;
size = s;
top_index = t;
stack_ptr = new T[size];
for (int i = 0; i <= top_index; i++) {
file >> stack_ptr[i];
}
file.close();
}
~Stack() {
delete[] stack_ptr;
}
};
int main() {
Stack<int> s(5);
s.push(1);
s.push(2);
s.push(3);
s.push(4);
s.push(5);
s.print();
s.pop();
s.pop();
s.print();
cout << "Peek: " << s.peek() << endl;
s[2] = 8;
s.print();
Stack<int> t;
t = s;
t.print();
s.saveToFile("stack.txt");
Stack<int> u;
u.readFromFile("stack.txt");
u.print();
return 0;
}
```
上述代码实现了一个模板类 `Stack<T>`,其中 `T` 是栈中元素的类型。类中包括了栈的基本操作,如入栈、出栈、判断栈是否为空、遍历栈、返回栈顶元素等等。同时,我们也重载了 `[]` 运算符和赋值运算符 `=`,支持文件输入输出,可以方便地保存和读取栈的状态。
相关推荐
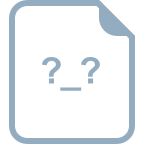
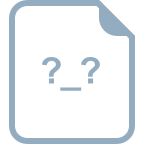
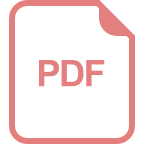
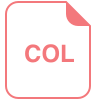
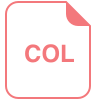
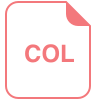
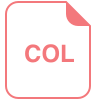
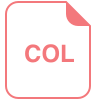









