def file_based_convert_examples_to_features( examples, label_list, max_seq_length, tokenizer, output_file):pytorch实现
时间: 2024-05-07 10:20:50 浏览: 166
这是一个 PyTorch 实现的函数,用于将输入的文本样本列表 `examples` 转换为特征并写入到输出文件 `output_file` 中。这个函数的输入参数包括:
- `examples`: 输入的文本样本列表;
- `label_list`: 标签列表;
- `max_seq_length`: 最大序列长度;
- `tokenizer`: 分词器;
- `output_file`: 输出文件路径。
下面是这个函数的实现代码:
```python
import torch
import numpy as np
def file_based_convert_examples_to_features(examples, label_list, max_seq_length, tokenizer, output_file):
writer = open(output_file, "w", encoding='utf-8')
for (ex_index, example) in enumerate(examples):
tokens_a = tokenizer.tokenize(example.text_a)
tokens_b = None
if example.text_b:
tokens_b = tokenizer.tokenize(example.text_b)
if tokens_b:
# 将句子 A 和句子 B 拼接起来
# 我们假设 [SEP] 是两个句子的分隔符
tokens = []
segment_ids = []
for token in tokens_a:
tokens.append(token)
segment_ids.append(0)
tokens.append("[SEP]")
segment_ids.append(0)
for token in tokens_b:
tokens.append(token)
segment_ids.append(1)
tokens.append("[SEP]")
segment_ids.append(1)
else:
# 如果只有一个句子,则只需要在前后加上 [CLS] 和 [SEP]
tokens = []
segment_ids = []
for token in tokens_a:
tokens.append(token)
segment_ids.append(0)
tokens.append("[SEP]")
segment_ids.append(0)
# 将 tokens 转换为对应的 id
input_ids = tokenizer.convert_tokens_to_ids(tokens)
# 将 input_ids 和 segment_ids 转换为 PyTorch 的 tensor
input_ids = torch.tensor(input_ids, dtype=torch.long)
segment_ids = torch.tensor(segment_ids, dtype=torch.long)
# 如果句子的长度小于 max_seq_length,则在后面补上 [PAD],直到达到 max_seq_length
input_mask = torch.ones(input_ids.size(), dtype=torch.long)
if input_ids.size(0) < max_seq_length:
padding = torch.ones(max_seq_length - input_ids.size(0), dtype=torch.long)
input_ids = torch.cat([input_ids, padding], dim=0)
input_mask = torch.cat([input_mask, padding], dim=0)
segment_ids_padding = torch.zeros(max_seq_length - input_ids.size(0), dtype=torch.long)
segment_ids = torch.cat([segment_ids, segment_ids_padding], dim=0)
assert input_ids.size() == torch.Size([max_seq_length])
assert input_mask.size() == torch.Size([max_seq_length])
assert segment_ids.size() == torch.Size([max_seq_length])
label_id = label_list.index(example.label)
label_id = torch.tensor(label_id, dtype=torch.long)
writer.write("{}\t{}\t{}\n".format(input_ids.numpy().tolist(),
input_mask.numpy().tolist(),
segment_ids.numpy().tolist(),
label_id.numpy().tolist()))
writer.close()
```
这个函数的主要思路是先将输入的文本样本进行分词,然后将分词后的结果转换为对应的 id,接着将句子拼接成一个输入序列,并在后面补上 [PAD],直到序列长度达到 `max_seq_length`。最后将输入序列、输入掩码、句子分隔符标记以及标签转换为 PyTorch 的 tensor,并写入到输出文件中。
阅读全文
相关推荐
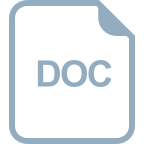
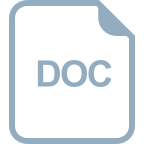
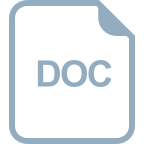

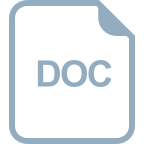
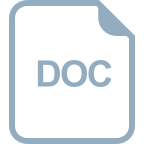
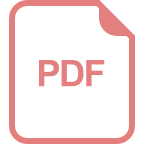
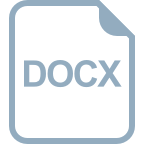
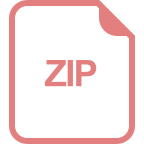
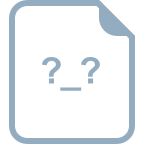
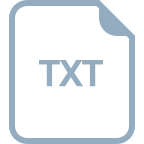
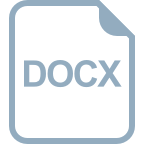
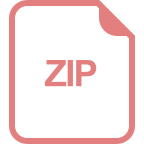
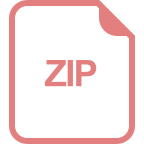
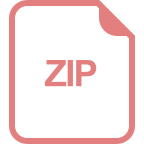