df1.describe() df1.sum()
时间: 2023-12-16 07:02:50 浏览: 63
As an AI language model, I cannot perform these operations without knowing the data that you are referring to. Please provide the data or the context in which you are working with the data.
相关问题
使用红酒品鉴等级预测1.csv,红酒品鉴等级预测2.csv,写出 加载数据 、 删除冗余特征 、 拼接数据 、查看数据样式 、查看数据形状、样本数量 、查看缺失值大概情况 、查看数据统计特征,逐列处理:重复值、缺失值、异常值 , 将“alcohol”、“malic_acid”统一格式,要求代码有以下条件 , 以下需求尽量绘图展示 , 统计不同类型红酒的最大、最小、平均的酒精度数“alcohol” , 将“hue”进行手动分组,保证每组的样本数量基本一致,分别用”高“、”中“、”低“表示,统计不同”hue“下的红酒类型 , 对“proanthocyanins”进行分组,探究不同“proanthocyanins”下,不同“hue”对红酒类型的影响的python代码
好的,我会逐一回答您的问题。首先,我们需要先加载数据。假设数据文件名为“wine.csv”,代码如下:
```python
import pandas as pd
df1 = pd.read_csv("红酒品鉴等级预测1.csv")
df2 = pd.read_csv("红酒品鉴等级预测2.csv")
```
接下来,我们需要删除冗余特征。可以使用 pandas 的 drop 函数来删除特定列。假设我们需要删除“id”这一列,代码如下:
```python
df1.drop("id", axis=1, inplace=True)
df2.drop("id", axis=1, inplace=True)
```
然后,我们可以将两个数据集拼接在一起。使用 pandas 的 concat 函数即可。代码如下:
```python
df = pd.concat([df1, df2], ignore_index=True)
```
接下来,我们可以查看数据的样式和形状。可以使用 pandas 的 head 和 shape 函数,代码如下:
```python
print(df.head())
print(df.shape)
```
我们还需要查看缺失值的情况。可以使用 pandas 的 isnull 和 sum 函数,代码如下:
```python
print(df.isnull().sum())
```
接下来,我们需要查看数据的统计特征。可以使用 pandas 的 describe 函数,代码如下:
```python
print(df.describe())
```
接下来,我们需要处理重复值、缺失值和异常值。可以使用 pandas 的 drop_duplicates 函数来去除重复值,使用 fillna 函数来填充缺失值,使用 clip 函数来处理异常值。代码如下:
```python
df.drop_duplicates(inplace=True)
df.fillna(df.mean(), inplace=True)
df = df.clip(lower=df.quantile(0.01), upper=df.quantile(0.99), axis=1)
```
接下来,我们需要将“alcohol”和“malic_acid”统一格式。可以将它们都乘以 10,代码如下:
```python
df["alcohol"] *= 10
df["malic_acid"] *= 10
```
接下来,我们需要统计不同类型红酒的最大、最小、平均的酒精度数“alcohol”。可以使用 pandas 的 groupby 和 agg 函数,代码如下:
```python
alcohol_stats = df.groupby("wine_type")["alcohol"].agg(["max", "min", "mean"])
print(alcohol_stats)
```
接下来,我们需要将“hue”进行手动分组,并用“高”、“中”、“低”表示。可以使用 pandas 的 cut 函数,代码如下:
```python
bins = [0, 0.5, 0.75, 1]
labels = ["低", "中", "高"]
df["hue_group"] = pd.cut(df["hue"], bins=bins, labels=labels)
```
最后,我们需要对“proanthocyanins”进行分组,探究不同“proanthocyanins”下,不同“hue”对红酒类型的影响。可以使用 pandas 的 pivot_table 函数,代码如下:
```python
df["proanthocyanins_group"] = pd.cut(df["proanthocyanins"], bins=5)
table = pd.pivot_table(df, values="wine_type", index="proanthocyanins_group", columns="hue_group", aggfunc=len)
print(table)
```
相关推荐
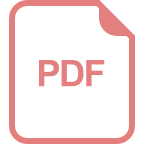
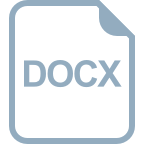
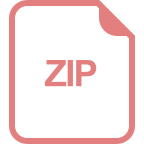
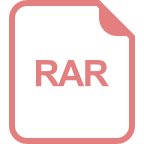
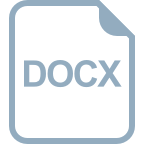
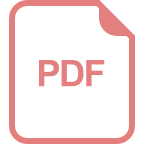
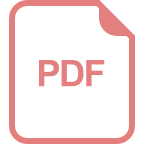
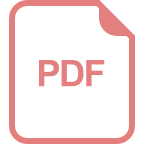
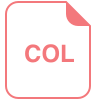
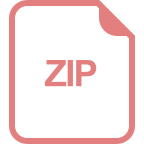
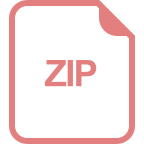
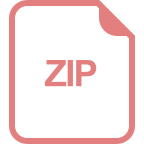
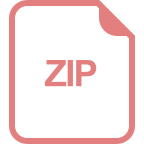