android 蓝牙连接代码
时间: 2023-07-26 07:03:27 浏览: 109
以下是 Android 中连接蓝牙设备的示例代码:
1. 在 AndroidManifest.xml 文件中添加蓝牙权限:
```xml
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
```
2. 在 Activity 中获取 BluetoothAdapter 实例并开启蓝牙:
```java
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter == null) {
// 设备不支持蓝牙
return;
}
if (!bluetoothAdapter.isEnabled()) {
// 若蓝牙未开启,则请求用户开启蓝牙
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
```
3. 搜索并连接蓝牙设备:
```java
// 获取已配对的设备列表
Set<BluetoothDevice> pairedDevices = bluetoothAdapter.getBondedDevices();
if (pairedDevices.size() > 0) {
// 遍历已配对的设备列表,查找指定设备
for (BluetoothDevice device : pairedDevices) {
if (device.getName().equals("MyDevice")) {
// 找到设备,开始连接
connectToDevice(device);
break;
}
}
} else {
// 没有已配对的设备,开始搜索新设备
startDiscovery();
}
// 注册广播接收器,监听搜索到的设备
private final BroadcastReceiver discoveryReceiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
// 获取搜索到的设备
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
// 判断是否为指定设备
if (device.getName().equals("MyDevice")) {
// 找到设备,停止搜索并开始连接
bluetoothAdapter.cancelDiscovery();
connectToDevice(device);
}
}
}
};
// 开始搜索新设备
private void startDiscovery() {
IntentFilter filter = new IntentFilter(BluetoothDevice.ACTION_FOUND);
registerReceiver(discoveryReceiver, filter); // 注册广播接收器
bluetoothAdapter.startDiscovery();
}
// 连接设备
private void connectToDevice(BluetoothDevice device) {
BluetoothSocket socket = null;
try {
socket = device.createRfcommSocketToServiceRecord(MY_UUID);
socket.connect();
} catch (IOException e) {
e.printStackTrace();
try {
socket.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
```
注意:上述代码中的 `MY_UUID` 为自定义的 UUID,用于标识蓝牙服务端与客户端之间的通信。在服务端代码中也需要使用相同的 UUID。
阅读全文
相关推荐
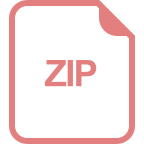
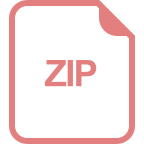
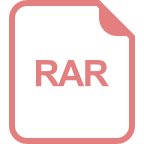
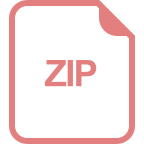
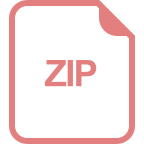
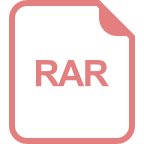
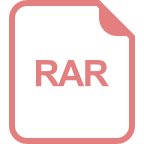
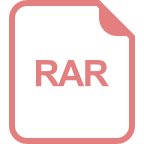
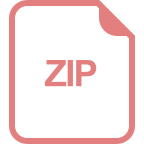
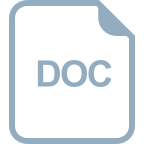




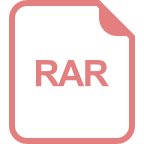