帮我写段多线程的c语言代码
时间: 2023-09-25 19:06:51 浏览: 90
### 回答1:
#include <stdio.h>
#include <pthread.h>
void *thread_function1(void *arg);
void *thread_function2(void *arg);
int main()
{
pthread_t thread1, thread2;
char *message1 = "Thread 1";
char *message2 = "Thread 2";
int ret1, ret2;
ret1 = pthread_create(&thread1, NULL, thread_function1, (void*) message1);
ret2 = pthread_create(&thread2, NULL, thread_function2, (void*) message2);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("线程1返回值:%d\n", ret1);
printf("线程2返回值:%d\n", ret2);
return 0;
}
void *thread_function1(void *arg)
{
printf("thread_function1 被调用,参数是:%s\n", (char *)arg);
pthread_exit(NULL);
}
void *thread_function2(void *arg)
{
printf("thread_function2 被调用,参数是:%s\n", (char *)arg);
pthread_exit(NULL);
}
### 回答2:
以下是一个简单的多线程的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
void *printHello(void *threadID) {
long tid;
tid = (long) threadID;
printf("Thread ID: %ld Hello, World!\n", tid);
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for(t=0; t<NUM_THREADS; t++) {
printf("Creating thread %ld\n", t);
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if(rc) {
printf("Error: unable to create thread, %d\n", rc);
exit(-1);
}
}
pthread_exit(NULL);
}
```
在上述代码中,首先包含了需要的头文件`stdio.h`、`stdlib.h`和`pthread.h`。然后定义了一个宏`NUM_THREADS`来表示线程的数量。
接下来,通过`void *printHello(void *threadID)`函数定义了线程的执行函数。这个函数将打印线程的ID并输出"Hello, World!"。最后,线程通过`pthread_exit(NULL)`函数退出。
在`main()`函数中,首先创建了一个`pthread_t`类型的数组`threads`,用于存放线程标识符。然后通过循环创建指定数量的线程,并调用`pthread_create()`函数创建线程。如果创建线程出现错误,则输出错误信息并通过`exit(-1)`函数退出程序。
最后,通过调用`pthread_exit(NULL)`函数等待所有线程结束后退出程序。
此段代码演示了如何使用多线程来实现同时执行多个任务的功能。
### 回答3:
下面是一个简单的多线程的C语言代码示例:
```c
#include <stdio.h>
#include <pthread.h>
// 线程函数1
void* thread_func1(void* arg) {
int i;
for(i = 0; i < 10; i++) {
printf("线程1: %d\n", i);
}
pthread_exit(NULL);
}
// 线程函数2
void* thread_func2(void* arg) {
int i;
for(i = 0; i < 10; i++) {
printf("线程2: %d\n", i);
}
pthread_exit(NULL);
}
int main() {
pthread_t tid1, tid2;
// 创建线程1
if(pthread_create(&tid1, NULL, thread_func1, NULL) != 0) {
fprintf(stderr, "无法创建线程1\n");
return 1;
}
// 创建线程2
if(pthread_create(&tid2, NULL, thread_func2, NULL) != 0) {
fprintf(stderr, "无法创建线程2\n");
return 1;
}
// 等待线程1结束
if(pthread_join(tid1, NULL) != 0) {
fprintf(stderr, "线程1加入失败\n");
return 1;
}
// 等待线程2结束
if(pthread_join(tid2, NULL) != 0) {
fprintf(stderr, "线程2加入失败\n");
return 1;
}
return 0;
}
```
这段代码创建了两个线程(线程1和线程2),每个线程分别打印数字0到9。主线程使用pthread_join函数等待两个子线程的结束,并在子线程结束后回收资源。在这个简单的例子中,两个线程的执行顺序是不确定的,可能会交替执行。
相关推荐
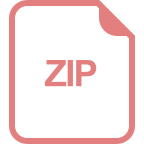
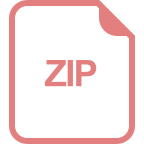
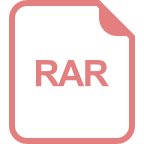
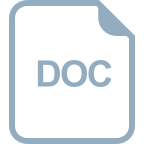
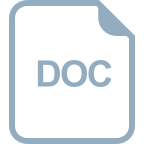
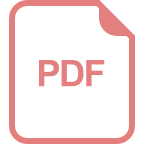
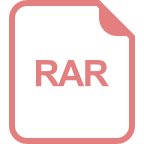
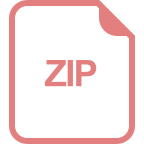
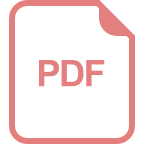
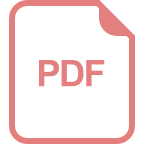
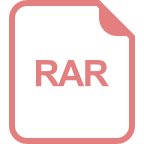
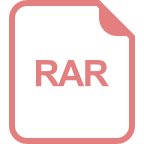
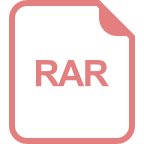
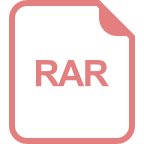
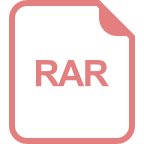
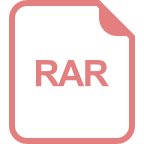