c#判断文件存在前还需要判断文件夹是否存在吗
时间: 2023-09-03 17:05:58 浏览: 146
在创建文件之前,需要先判断文件所在的文件夹是否存在。如果文件夹不存在,需要先创建文件夹,再创建文件。因此,在创建文件之前,需要先进行文件夹是否存在的判断。可以使用C#中的Directory类实现此功能。具体代码如下:
```csharp
using System.IO;
string folderPath = @"C:\example\folder"; // 文件夹路径
string filePath = @"C:\example\folder\file.txt"; // 文件路径
if (!Directory.Exists(folderPath)) // 判断文件夹是否存在
{
Directory.CreateDirectory(folderPath); // 创建文件夹
}
if (!File.Exists(filePath)) // 判断文件是否存在
{
File.Create(filePath); // 创建文件
}
```
其中,先对文件夹进行判断,如果不存在则调用Directory.CreateDirectory()方法创建文件夹。然后再对文件进行判断,如果不存在则调用File.Create()方法创建文件。
相关问题
C# 判断文件夹下是否存在文件
C is a programming language that was developed in the early 1970s by Dennis Ritchie at Bell Labs. It is a general-purpose, procedural programming language that is widely used in the development of operating systems, embedded systems, and other high-performance applications.
C is a low-level language, meaning that it provides direct access to the computer's hardware and memory, which makes it a powerful tool for systems programming. It is also a compiled language, which means that the code written in C is translated into machine code before it can be executed.
Some of the key features of C include its simplicity, efficiency, and portability. It has a relatively small set of keywords and constructs, which makes it easy to learn and use. It also has excellent performance and is able to run on a wide range of platforms, from small embedded devices to large mainframe computers.
C has had a huge impact on the field of computer science and has influenced many other programming languages, including C++, Java, and Python. It is still widely used today, particularly in the development of operating systems, device drivers, and other low-level software.
C# 如何判断文件的文件夹是否存在,不存在就新增存放文件的文件夹
在 C# 中,你可以使用 `DirectoryInfo` 和 `Directory.CreateDirectory` 方法来判断和创建文件夹。首先,你需要获取到文件夹路径,然后检查其是否存在。如果不存在,就新建目录。以下是示例代码:
```csharp
using System.IO;
string folderPath = @"C:\YourFolderPath\NewFolder"; // 指定的文件夹路径
// 检查文件夹是否存在
var directoryInfo = new DirectoryInfo(folderPath);
if (!directoryInfo.Exists)
{
// 文件夹不存在,尝试创建新的文件夹
try
{
directoryInfo.Create();
Console.WriteLine("文件夹已创建");
}
catch (UnauthorizedAccessException e)
{
Console.WriteLine($"权限不足,无法创建文件夹:{e.Message}");
}
catch (IOException e)
{
Console.WriteLine($"无法创建文件夹:{e.Message}");
}
}
else
{
Console.WriteLine($"文件夹 '{folderPath}' 已存在");
}
```
这段代码首先通过 `DirectoryInfo` 构造函数创建对指定路径的表示,然后检查是否存在。如果不存在,尝试创建并捕获可能出现的 `UnauthorizedAccessException`(权限问题)或 `IOException`(IO操作失败)。如果文件夹已经存在,它将直接告知用户。
阅读全文
相关推荐






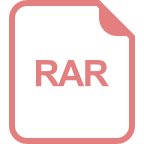









