import pandas as pd import matplotlib.pyplot as plt import seaborn as sns from sklearn.model_selection import train_test_split from sklearn.tree import DecisionTreeClassifier from sklearn.metrics import accuracy_score, confusion_matrix # 读取Excel文件 df = pd.read_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测样本.xlsx') # 提取特征和标签 X = df[['高程', '起伏度', '桥梁长', '道路长', '平均坡度', '平均地温', 'T小于0', '相态']] y = df['交通风险'] # 划分训练集和验证集 X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # 创建决策树模型 clf = DecisionTreeClassifier() # 使用训练集拟合模型 clf.fit(X_train, y_train) # 预测验证集的标签 y_pred = clf.predict(X_test) # 计算模型的准确率 accuracy = accuracy_score(y_test, y_pred) # 输出模型的准确率 print('Accuracy:', accuracy) # 输出混淆矩阵 cm = confusion_matrix(y_test, y_pred) plt.figure(figsize=(6,6)) sns.heatmap(cm, annot=True, cmap='Blues') plt.xlabel('Predicted label') plt.ylabel('True label') plt.title('Confusion Matrix') plt.savefig('E:/桌面/预测脆弱性/20230523/预测样本/预测结果/决策树confusion_matrix.png') # 读取新的Excel数据 new_data = pd.read_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测结果/交通风险预测096.xlsx') # 提取特征 X_new = new_data[['高程', '起伏度', '桥梁长', '道路长', '平均坡度', '平均地温', 'T小于0', '相态']] # 预测新数据的标签 y_new = clf.predict(X_new) # 将预测结果输出到新的Excel文件中 new_data['交通风险预测结果'] = y_new new_data.to_excel('E:/桌面/预测脆弱性/20230523/预测样本/预测结果/交通风险预测096-决策树结果.xlsx', index=False)修改代码输出混淆矩阵
时间: 2023-08-15 09:04:56 浏览: 41
将以下代码:
```
plt.figure(figsize=(6,6))
sns.heatmap(cm, annot=True, cmap='Blues')
plt.xlabel('Predicted label')
plt.ylabel('True label')
plt.title('Confusion Matrix')
plt.savefig('E:/桌面/预测脆弱性/20230523/预测样本/预测结果/决策树confusion_matrix.png')
```
修改为:
```
plt.figure(figsize=(6,6))
sns.heatmap(cm, annot=True, cmap='Blues')
plt.xlabel('Predicted label')
plt.ylabel('True label')
plt.title('Confusion Matrix')
plt.show()
```
运行后即可在控制台中输出混淆矩阵。
相关问题
import pandas as pd import numpy as np import matplotlib.pyplot as plt import seaborn as sns from sklearn import tree
以下是使用import语句导入pandas、numpy、matplotlib.pyplot、seaborn和sklearn.tree的示例代码:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn import tree
```
- pandas是一个数据处理库,用于读取、处理和分析数据。
- numpy是一个数学库,用于处理数组和矩阵等数学运算。
- matplotlib.pyplot是一个绘图库,用于绘制各种类型的图表。
- seaborn是一个基于matplotlib的数据可视化库,提供了更高级别的界面和更多的图表类型。
- sklearn.tree是scikit-learn库中的一个模块,用于实现决策树算法。
from sklearn.datasets import load_boston import numpy as np import pandas as pd import matplotlib.pyplot as plt from sklearn.linear_model import LassoCV import seaborn as sns from sklearn.preprocessing import StandardScaler from sklearn.model_selection import train_test_split
这段代码主要是导入了一些Python的第三方库,包括:
- sklearn.datasets:用于加载波士顿房价数据集。
- numpy:用于进行科学计算,如数组操作、数值计算等。
- pandas:用于数据处理和分析,如数据读取、转换、合并等。
- matplotlib.pyplot:用于绘制数据可视化图表,如折线图、散点图等。
- sklearn.linear_model:用于线性回归模型的构建和训练。
- seaborn:基于matplotlib的数据可视化库,提供更加美观的图表风格。
- sklearn.preprocessing:用于数据预处理,如标准化、归一化、缺失值处理等。
- sklearn.model_selection:用于模型训练和评估的数据集划分、交叉验证等操作。
其中,load_boston函数用于加载波士顿房价数据集,LassoCV是一种线性回归模型,可以进行自动特征选择和正则化,StandardScaler用于对数据进行标准化处理,train_test_split用于将数据集划分为训练集和测试集。
相关推荐
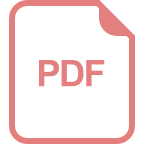
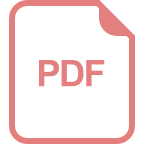
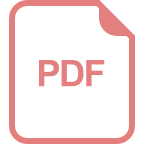













