用C语言实现一个学生管理系统 要求包括学生姓名 性别 学号 班级 高数成绩 英语成绩 C语言成绩 总成绩
时间: 2023-06-12 12:07:43 浏览: 196
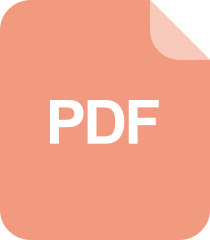
C语言结构体版学生成绩管理系统
以下是一个简单的学生管理系统的 C 语言代码实现,包括学生姓名、性别、学号、班级、高数成绩、英语成绩、C 语言成绩和总成绩的管理:
```c
#include <stdio.h>
struct student {
char name[20];
char gender[10];
int id;
int class;
float math_score;
float english_score;
float c_score;
float total_score;
};
int main()
{
struct student stu[50];
int num, i;
float total_math_score = 0, total_english_score = 0, total_c_score = 0;
printf("请输入学生数:");
scanf("%d", &num);
for (i = 0; i < num; i++) {
printf("\n请输入第 %d 个学生的信息:\n", i + 1);
printf("姓名:");
scanf("%s", stu[i].name);
printf("性别:");
scanf("%s", stu[i].gender);
printf("学号:");
scanf("%d", &stu[i].id);
printf("班级:");
scanf("%d", &stu[i].class);
printf("高数成绩:");
scanf("%f", &stu[i].math_score);
printf("英语成绩:");
scanf("%f", &stu[i].english_score);
printf("C语言成绩:");
scanf("%f", &stu[i].c_score);
stu[i].total_score = stu[i].math_score + stu[i].english_score + stu[i].c_score;
total_math_score += stu[i].math_score;
total_english_score += stu[i].english_score;
total_c_score += stu[i].c_score;
}
printf("\n学生信息如下:\n");
printf("姓名\t性别\t学号\t班级\t高数成绩\t英语成绩\tC语言成绩\t总成绩\n");
for (i = 0; i < num; i++) {
printf("%s\t%s\t%d\t%d\t%.2f\t\t%.2f\t\t%.2f\t\t%.2f\n", stu[i].name, stu[i].gender, stu[i].id, stu[i].class, stu[i].math_score, stu[i].english_score, stu[i].c_score, stu[i].total_score);
}
printf("平均分\t\t\t\t\t%.2f\t\t%.2f\t\t%.2f\n", total_math_score / num, total_english_score / num, total_c_score / num);
return 0;
}
```
该程序中使用了一个结构体来存储每个学生的信息,通过循环来输入和输出学生信息,并计算每个学生的总成绩和所有学生的平均分。
阅读全文
相关推荐
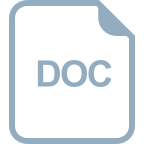
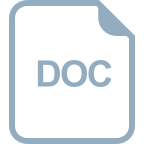



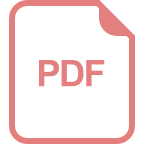
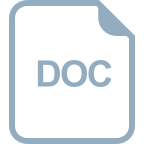








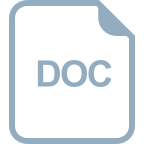
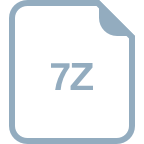