创建一个学生类(包括姓名,年龄,班级,密码),创建若干该类的对象并保存到文件中(密码不保存:),从文件读取对象后显示在屏幕上,Java编程)
时间: 2023-11-27 19:03:06 浏览: 74
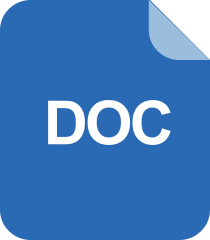
Java面向对象编程初步(1)
好的,这是一个简单的实现:
```java
import java.io.*;
class Student implements Serializable {
private String name;
private int age;
private String className;
private transient String password;
public Student(String name, int age, String className, String password) {
this.name = name;
this.age = age;
this.className = className;
this.password = password;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getClassName() {
return className;
}
public String getPassword() {
return password;
}
public String toString() {
return "Name: " + name + "\nAge: " + age + "\nClass: " + className;
}
}
public class Main {
public static void main(String[] args) {
Student s1 = new Student("John", 18, "Class A", "password123");
Student s2 = new Student("Mary", 19, "Class B", "password456");
try {
// Save objects to file
FileOutputStream fileOut = new FileOutputStream("students.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(s1);
out.writeObject(s2);
out.close();
fileOut.close();
System.out.println("Students saved to file.");
// Read objects from file
FileInputStream fileIn = new FileInputStream("students.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Student s3 = (Student) in.readObject();
Student s4 = (Student) in.readObject();
in.close();
fileIn.close();
// Print objects to screen
System.out.println("Student 1:");
System.out.println(s3);
System.out.println("\nStudent 2:");
System.out.println(s4);
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
这个程序创建了一个`Student`类,其中包括姓名、年龄、班级和密码等信息。然后,它创建了两个`Student`对象`s1`和`s2`,并将它们保存到名为`students.ser`的文件中。它然后再从文件中读取这些对象,并将它们打印到屏幕上。
注意,`password`属性被标记为`transient`,这意味着它不会被序列化到文件中。这是为了保护学生的隐私,以避免密码泄露。
阅读全文
相关推荐
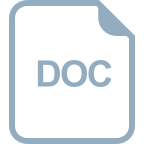
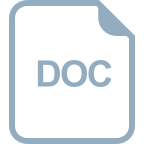
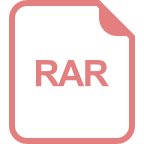
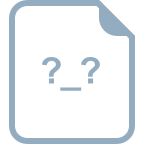
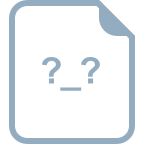
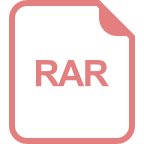
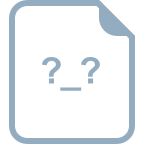
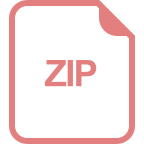
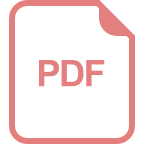
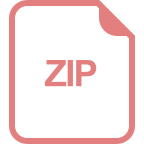
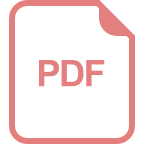
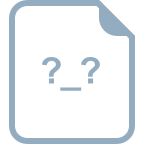
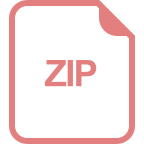
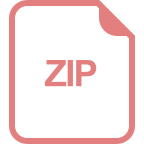
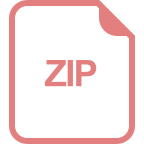