用Java写一个图书借阅管理系统
时间: 2023-11-09 14:08:44 浏览: 44
好的,我可以为您提供一个简单的图书借阅管理系统的Java代码。这个系统包括以下功能:
1. 添加图书
2. 删除图书
3. 查询图书
4. 借阅图书
5. 归还图书
首先,我们需要定义一个`Book`类,用来表示一本图书的信息,例如书名、作者、出版社、ISBN等:
```java
public class Book {
private String title;
private String author;
private String publisher;
private String isbn;
private boolean borrowed;
public Book(String title, String author, String publisher, String isbn) {
this.title = title;
this.author = author;
this.publisher = publisher;
this.isbn = isbn;
this.borrowed = false;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
public String getIsbn() {
return isbn;
}
public boolean isBorrowed() {
return borrowed;
}
public void setBorrowed(boolean borrowed) {
this.borrowed = borrowed;
}
}
```
然后,我们需要一个`Library`类,用来管理图书的借阅:
```java
import java.util.ArrayList;
import java.util.List;
public class Library {
private List<Book> books;
public Library() {
this.books = new ArrayList<>();
}
public void addBook(Book book) {
books.add(book);
}
public void removeBook(Book book) {
books.remove(book);
}
public List<Book> searchBooks(String keyword) {
List<Book> result = new ArrayList<>();
for (Book book : books) {
if (book.getTitle().contains(keyword) || book.getAuthor().contains(keyword) || book.getPublisher().contains(keyword) || book.getIsbn().contains(keyword)) {
result.add(book);
}
}
return result;
}
public void borrowBook(Book book) {
if (!book.isBorrowed()) {
book.setBorrowed(true);
} else {
System.out.println("This book is already borrowed.");
}
}
public void returnBook(Book book) {
if (book.isBorrowed()) {
book.setBorrowed(false);
} else {
System.out.println("This book is not borrowed.");
}
}
}
```
最后,我们可以编写一个`Main`类,用来测试上面的代码:
```java
import java.util.List;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Library library = new Library();
Book book1 = new Book("Java Tutorial", "John Smith", "Oracle", "1234567890");
Book book2 = new Book("Python for Beginners", "Jane Doe", "O'Reilly Media", "0987654321");
Book book3 = new Book("JavaScript Cookbook", "Bob Johnson", "Wiley", "1357908642");
library.addBook(book1);
library.addBook(book2);
library.addBook(book3);
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Welcome to the Library Management System!");
System.out.println("1. Add book");
System.out.println("2. Remove book");
System.out.println("3. Search book");
System.out.println("4. Borrow book");
System.out.println("5. Return book");
System.out.println("0. Exit");
System.out.print("Please enter your choice: ");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
System.out.print("Please enter the title of the book: ");
String title = scanner.nextLine();
System.out.print("Please enter the author of the book: ");
String author = scanner.nextLine();
System.out.print("Please enter the publisher of the book: ");
String publisher = scanner.nextLine();
System.out.print("Please enter the ISBN of the book: ");
String isbn = scanner.nextLine();
Book book = new Book(title, author, publisher, isbn);
library.addBook(book);
System.out.println("Book added successfully.");
break;
case 2:
System.out.print("Please enter the ISBN of the book: ");
isbn = scanner.nextLine();
List<Book> searchResult = library.searchBooks(isbn);
if (searchResult.size() == 1) {
Book bookToRemove = searchResult.get(0);
library.removeBook(bookToRemove);
System.out.println("Book removed successfully.");
} else {
System.out.println("Book not found.");
}
break;
case 3:
System.out.print("Please enter the keyword to search: ");
String keyword = scanner.nextLine();
List<Book> searchResult2 = library.searchBooks(keyword);
if (searchResult2.size() > 0) {
System.out.println("Search result:");
for (Book b : searchResult2) {
System.out.println(b.getTitle() + " by " + b.getAuthor() + " (" + b.getPublisher() + ", " + b.getIsbn() + ")");
}
} else {
System.out.println("No book found.");
}
break;
case 4:
System.out.print("Please enter the ISBN of the book: ");
isbn = scanner.nextLine();
List<Book> searchResult3 = library.searchBooks(isbn);
if (searchResult3.size() == 1) {
Book bookToBorrow = searchResult3.get(0);
library.borrowBook(bookToBorrow);
System.out.println("Book borrowed successfully.");
} else {
System.out.println("Book not found.");
}
break;
case 5:
System.out.print("Please enter the ISBN of the book: ");
isbn = scanner.nextLine();
List<Book> searchResult4 = library.searchBooks(isbn);
if (searchResult4.size() == 1) {
Book bookToReturn = searchResult4.get(0);
library.returnBook(bookToReturn);
System.out.println("Book returned successfully.");
} else {
System.out.println("Book not found.");
}
break;
case 0:
System.out.println("Goodbye!");
return;
default:
System.out.println("Invalid choice.");
break;
}
System.out.println();
}
}
}
```
这是一个简单的图书借阅管理系统,您可以根据需要进行修改和扩展。
相关推荐
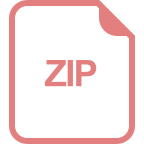
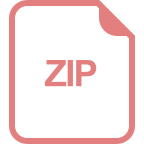
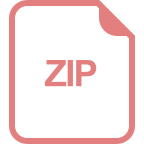
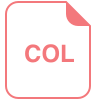
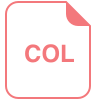
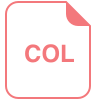
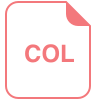
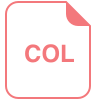









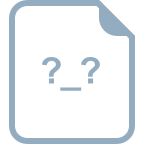