遗传算法优化神经网络的权值的代码
时间: 2023-09-03 18:11:05 浏览: 67
以下是使用遗传算法优化神经网络权值的示例代码:
首先,需要定义适应度函数,这个函数将评估每个个体的适应度,即神经网络的性能。在这个示例中,适应度函数将计算神经网络在给定数据集上的准确度。
```python
def fitness_function(weights):
# 使用权重更新网络
neural_network.set_weights(weights)
# 计算网络在数据集上的准确度
accuracy = calculate_accuracy(neural_network, dataset)
return accuracy
```
接下来,需要实现遗传算法的主要逻辑。这个示例中使用的遗传算法包括选择、交叉和变异三个操作。实现中使用了 Python 的 DEAP 库来简化遗传算法的实现。
```python
import random
from deap import base, creator, tools
# 定义遗传算法的参数
POPULATION_SIZE = 100
P_CROSSOVER = 0.9 # 交叉概率
P_MUTATION = 0.1 # 变异概率
MAX_GENERATIONS = 50
# 定义遗传算法中使用的对象
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox = base.Toolbox()
toolbox.register("attr_float", random.uniform, -1, 1)
toolbox.register("individual", tools.initRepeat, creator.Individual,
toolbox.attr_float, n=len(neural_network.get_weights()))
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
def cxTwoPointCopy(ind1, ind2):
"""交叉操作"""
size = min(len(ind1), len(ind2))
cxpoint1 = random.randint(0, size)
cxpoint2 = random.randint(0, size - 1)
if cxpoint2 >= cxpoint1:
cxpoint2 += 1
else:
cxpoint1, cxpoint2 = cxpoint2, cxpoint1
ind1[cxpoint1:cxpoint2], ind2[cxpoint1:cxpoint2] = ind2[cxpoint1:cxpoint2], ind1[cxpoint1:cxpoint2]
return ind1, ind2
def mutGaussian(individual, mu, sigma, indpb):
"""变异操作"""
size = len(individual)
for i in range(size):
if random.random() < indpb:
individual[i] += random.gauss(mu, sigma)
return individual,
toolbox.register("evaluate", fitness_function)
toolbox.register("mate", cxTwoPointCopy)
toolbox.register("mutate", mutGaussian, mu=0, sigma=0.2, indpb=0.1)
toolbox.register("select", tools.selTournament, tournsize=3)
# 运行遗传算法
population = toolbox.population(n=POPULATION_SIZE)
for generation in range(MAX_GENERATIONS):
offspring = algorithms.varAnd(population, toolbox, cxpb=P_CROSSOVER, mutpb=P_MUTATION)
fits = toolbox.map(toolbox.evaluate, offspring)
for fit, ind in zip(fits, offspring):
ind.fitness.values = fit
population = toolbox.select(offspring, k=len(population))
best_individual = tools.selBest(population, k=1)[0]
best_weights = best_individual
```
最后,将得到的最优权重应用到神经网络中,并测试其性能。
```python
neural_network.set_weights(best_weights)
test_accuracy = calculate_accuracy(neural_network, test_dataset)
print("Test accuracy:", test_accuracy)
```
相关推荐
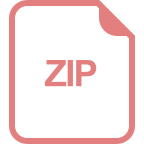
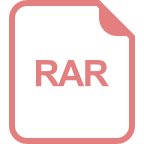
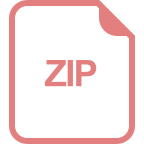














