使用JAVA来实现Flowable动态表单
时间: 2024-05-07 08:15:50 浏览: 18
Flowable动态表单是指根据不同的业务需求,动态生成表单。在JAVA中,可以使用以下步骤来实现Flowable动态表单:
1. 创建表单模型
首先,需要定义表单模型,包括表单名称、表单字段等。可以使用以下代码创建一个表单模型的Java类:
```
public class FormModel {
private String name;
private List<FormField> fields;
// getters and setters
}
public class FormField {
private String name;
private String type;
private String label;
// getters and setters
}
```
2. 创建表单控制器
接下来,需要创建一个控制器来处理表单的创建和提交。可以使用以下代码来创建一个简单的表单控制器:
```
@RestController
@RequestMapping("/forms")
public class FormController {
@PostMapping
public void createForm(@RequestBody FormModel form) {
// create form in Flowable
}
@PostMapping("/{formId}/submit")
public void submitForm(@PathVariable String formId, @RequestBody Map<String, Object> formValues) {
// submit form in Flowable
}
}
```
3. 使用Flowable表单服务创建表单
接下来,在createForm方法中,可以使用Flowable表单服务来创建表单。可以使用以下代码:
```
@Autowired
private FormService formService;
@PostMapping
public void createForm(@RequestBody FormModel form) {
StartFormData formData = formService.getStartFormData(processDefinitionId, form.getName());
List<FormProperty> formProperties = formData.getFormProperties();
for (FormField field : form.getFields()) {
formProperties.add(new FormPropertyImpl(field.getName(), field.getType(), field.getLabel(), null, null, null));
}
formService.submitStartFormData(processDefinitionId, formProperties);
}
```
4. 提交表单并保存数据
最后,在submitForm方法中,可以使用Flowable表单服务来提交表单并保存数据。可以使用以下代码:
```
@Autowired
private FormService formService;
@Autowired
private RuntimeService runtimeService;
@PostMapping("/{formId}/submit")
public void submitForm(@PathVariable String formId, @RequestBody Map<String, Object> formValues) {
Task task = taskService.createTaskQuery().processInstanceId(processInstanceId).singleResult();
TaskFormData taskFormData = formService.getTaskFormData(task.getId());
for (FormField field : form.getFields()) {
taskFormData.getFormProperties().add(new FormPropertyImpl(field.getName(), field.getType(), field.getLabel(), null, null, null));
}
formService.submitTaskFormData(task.getId(), formValues);
runtimeService.setVariable(task.getProcessInstanceId(), "formData", formValues);
}
```
这样,就可以使用JAVA来实现Flowable动态表单了。
相关推荐
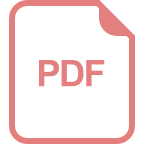
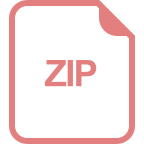
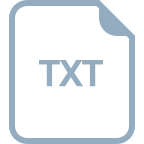














