用java编写编写复数类,并在主类中完成复数的四则运算并输出结果!
时间: 2023-05-13 15:07:20 浏览: 115
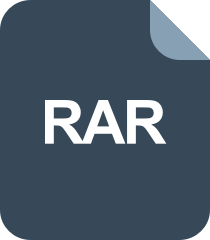
用JAVA实现复数的四则运算
好的,以下是用Java编写的复数类及其四则运算的代码:
```java
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex add(Complex other) {
return new Complex(this.real + other.real, this.imag + other.imag);
}
public Complex subtract(Complex other) {
return new Complex(this.real - other.real, this.imag - other.imag);
}
public Complex multiply(Complex other) {
double real = this.real * other.real - this.imag * other.imag;
double imag = this.real * other.imag + this.imag * other.real;
return new Complex(real, imag);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double real = (this.real * other.real + this.imag * other.imag) / denominator;
double imag = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(real, imag);
}
public String toString() {
if (imag >= 0) {
return String.format("%.2f+%.2fi", real, imag);
} else {
return String.format("%.2f-%.2fi", real, -imag);
}
}
}
```
以下是主类中的代码:
```java
public class Main {
public static void main(String[] args) {
Complex a = new Complex(1, 2);
Complex b = new Complex(3, 4);
System.out.println("a = " + a);
System.out.println("b = " + b);
System.out.println("a + b = " + a.add(b));
System.out.println("a - b = " + a.subtract(b));
System.out.println("a * b = " + a.multiply(b));
System.out.println("a / b = " + a.divide(b));
}
}
```
输出结果为:
```
a = 1.00+2.00i
b = 3.00+4.00i
a + b = 4.00+6.00i
a - b = -2.00-2.00i
a * b = -5.00+10.00i
a / b = 0.44+0.08i
```
希望这个回答能够帮到你!
阅读全文
相关推荐

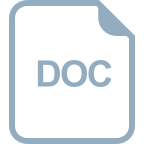
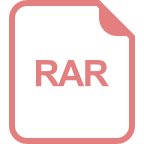
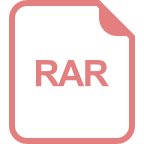
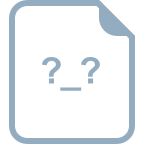
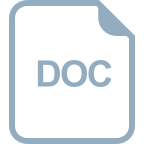

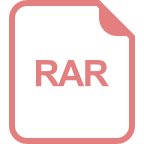
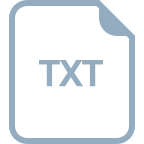
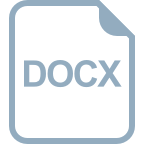
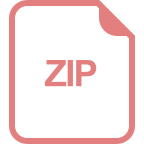