请建立一个对象数组,内放5个学生数据(学号,姓名,成绩),构造一个函数max,传入对象数组作为参数,在max函数中中找出5个学生中成绩最高者,并输出其学号,姓名,主函数示例代码: int main(int argc, char *argv[]) { Student stu[5]; string name,no; float score; for(int i=0;i<5;i++) { cin>>no>>name>>score; stu[i].setNo(no); stu[i].setName(name); stu[i].setScore(score); } Student temp=max(stu); temp.display(); }
时间: 2024-03-07 10:52:26 浏览: 68
好的,下面是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string no;
string name;
float score;
public:
void setNo(string n) {
no = n;
}
void setName(string n) {
name = n;
}
void setScore(float s) {
score = s;
}
string getNo() {
return no;
}
string getName() {
return name;
}
float getScore() {
return score;
}
void display() {
cout << "学号:" << no << " 姓名:" << name << " 成绩:" << score << endl;
}
};
Student max(Student stu[]) {
int maxIndex = 0;
for (int i = 1; i < 5; i++) {
if (stu[i].getScore() > stu[maxIndex].getScore()) {
maxIndex = i;
}
}
return stu[maxIndex];
}
int main(int argc, char *argv[]) {
Student stu[5];
string name, no;
float score;
for (int i = 0; i < 5; i++) {
cin >> no >> name >> score;
stu[i].setNo(no);
stu[i].setName(name);
stu[i].setScore(score);
}
Student temp = max(stu);
temp.display();
return 0;
}
```
在这个程序中,我们首先定义了一个 `Student` 类,包含学号、姓名和成绩三个属性,以及相应的 set 和 get 函数和一个 `display` 函数用于输出信息。
接着我们定义了一个 `max` 函数,用于找出学生数组中成绩最高的学生,最终返回该学生对象。在 `main` 函数中,我们创建了一个包含 5 个学生对象的数组,通过循环输入每个学生的信息,然后调用 `max` 函数找出成绩最高者,并使用该学生对象的 `display` 函数输出信息。
希望这个程序可以帮助到你!
阅读全文
相关推荐
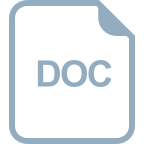
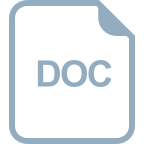
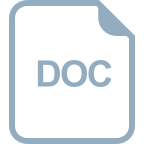


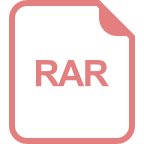












