在写成员变量的时候,为什么super调用的父类成员变量只能写在等号右边
时间: 2024-05-01 10:23:53 浏览: 50
在子类中,我们可以通过 `super` 关键字来调用父类中的成员变量。但是,我们只能在等号右边给父类成员变量赋值,而不能在等号左边。
这是因为在 Java 中,成员变量的初始化是在构造方法之前完成的。在子类的构造方法中,如果我们试图给父类成员变量赋值,这些成员变量实际上已经被初始化了。因此,我们只能在等号右边给父类成员变量赋值,而不能在等号左边。
例如,假设我们有一个父类 `Animal` 和一个子类 `Cat`:
``` java
public class Animal {
protected int age;
}
public class Cat extends Animal {
public Cat(int age) {
super.age = age; // 合法
// age = super.age; // 非法,会导致编译错误
}
}
```
在 `Cat` 的构造方法中,我们可以使用 `super.age = age` 来给父类成员变量赋值。但是,如果我们尝试使用 `age = super.age`,就会导致编译错误。
相关问题
1)所有类省略成员变量的修改器和读取器; (2)GeometricObject类的color、isFilled、createdDate属性分别表示图形的颜色、是否填充和创建日期; (3)GeometricObject类的toString()方法,返回描述图形颜色、是否填充信息的字符串(不需要返回创建时间); (4)Rectangle类width和heigth属性分别表示矩形的宽和高; (5)Rectangle类的toString()方法返回描述矩形的颜色、是否填充、半径、面积信息(getArea()方法实现),注意调用父类的toString()方法获取矩形的颜色和是否填充信息; (6)Rectangle类的equals方法用于判断两个矩形对象是否相等,判断依据:如果两个矩形的面积相等,则二者相等; (7)Circle类中的toString()方法返回描述圆的颜色、是否填充、半径、面积信息(getArea()方法实现),注意调用父类的toString()方法获取圆的颜色和是否填充信息; (8)Circle类的equals方法用于判断两个圆对象是否相等,判断依据:如果两个圆的半径相等,则二者相等; (9)Color类的三个成员变量red、green、blue,分别表示颜色中红绿蓝三原色的透明度值,三者的取值范围为[0.0 - 1.0],带下划线部分表示静态常量,等号右侧给出了三原色的透明度值; (10)Color类的equals()方法用于判断两个颜色对象是否相等,判断依据:如果两个颜色的三原色透明度值相等,则二者相等; (11)Color类的toString()方法,用于返回描述颜色的字符串。
下面是根据题目要求编写的GeometricObject、Rectangle、Circle和Color类的代码:
GeometricObject类:
```java
import java.util.Date;
public class GeometricObject {
private String color;
private boolean isFilled;
private Date createdDate;
public GeometricObject() {
createdDate = new Date();
}
public GeometricObject(String color, boolean isFilled) {
this.color = color;
this.isFilled = isFilled;
createdDate = new Date();
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public boolean isFilled() {
return isFilled;
}
public void setFilled(boolean filled) {
isFilled = filled;
}
public Date getCreatedDate() {
return createdDate;
}
public void setCreatedDate(Date createdDate) {
this.createdDate = createdDate;
}
public String toString() {
return "color: " + color + ", filled: " + isFilled;
}
}
```
Rectangle类:
```java
public class Rectangle extends GeometricObject {
private double width;
private double height;
public Rectangle() {
}
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public Rectangle(double width, double height, String color, boolean isFilled) {
super(color, isFilled);
this.width = width;
this.height = height;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getArea() {
return width * height;
}
public String toString() {
return super.toString() + ", width: " + width + ", height: " + height + ", area: " + getArea();
}
public boolean equals(Rectangle rectangle) {
return getArea() == rectangle.getArea();
}
}
```
Circle类:
```java
public class Circle extends GeometricObject {
private double radius;
public Circle() {
}
public Circle(double radius) {
this.radius = radius;
}
public Circle(double radius, String color, boolean isFilled) {
super(color, isFilled);
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public String toString() {
return super.toString() + ", radius: " + radius + ", area: " + getArea();
}
public boolean equals(Circle circle) {
return radius == circle.getRadius();
}
}
```
Color类:
```java
public class Color {
public static final double RED = 1.0;
public static final double GREEN = 1.0;
public static final double BLUE = 1.0;
private double red;
private double green;
private double blue;
public Color() {
red = RED;
green = GREEN;
blue = BLUE;
}
public Color(double red, double green, double blue) {
this.red = red;
this.green = green;
this.blue = blue;
}
public double getRed() {
return red;
}
public void setRed(double red) {
this.red = red;
}
public double getGreen() {
return green;
}
public void setGreen(double green) {
this.green = green;
}
public double getBlue() {
return blue;
}
public void setBlue(double blue) {
this.blue = blue;
}
public boolean equals(Color color) {
return red == color.getRed() && green == color.getGreen() && blue == color.getBlue();
}
public String toString() {
return "Red: " + red + ", Green: " + green + ", Blue: " + blue;
}
}
```
帮我用Java语言写一份实现一个简易计算器的代码,要求如下: (1)输入:不含变量的数学表达式的中缀形式,可以接受的操作符包括+、-、*、/、%和()以及小数点。 (2)输出:如果表达式正确,则输出表达式的结果,如果表达式非法,则输出错误信息。 (3)能够正确运算带括号的数学表达式的中缀形式。 (4)可以借助栈来实现,使用图形用户界面。
以下是一个简单的Java代码实现一个基础计算器,支持加、减、乘、除、取模和括号运算:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Calculator extends JFrame implements ActionListener {
private JTextField displayTextField;
public Calculator() {
super("简易计算器");
JPanel mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
displayTextField = new JTextField();
displayTextField.setEditable(false);
mainPanel.add(displayTextField, BorderLayout.NORTH);
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(4, 4));
String[] buttonLabels = {"7", "8", "9", "/", "4", "5", "6", "*", "1", "2", "3", "-", "0", ".", "=", "+"};
for (int i = 0; i < buttonLabels.length; i++) {
JButton button = new JButton(buttonLabels[i]);
button.addActionListener(this);
buttonPanel.add(button);
}
mainPanel.add(buttonPanel, BorderLayout.CENTER);
setContentPane(mainPanel);
}
private String calculate(String expression) {
// Check if expression is null or empty
if (expression == null || expression.isEmpty()) {
return "错误:表达式为空";
}
// Check if expression contains only valid characters
if (!expression.matches("[0-9+\\-*/%.()]+")) {
return "错误:表达式包含无效字符";
}
// Check if expression has balanced parentheses
int parenthesesCount = 0;
for (int i = 0; i < expression.length(); i++) {
char c = expression.charAt(i);
if (c == '(') {
parenthesesCount++;
} else if (c == ')') {
parenthesesCount--;
}
if (parenthesesCount < 0) {
return "错误:表达式包含未匹配的右括号";
}
}
if (parenthesesCount > 0) {
return "错误:表达式包含未匹配的左括号";
}
// Evaluate expression
try {
double result = evaluate(expression);
return Double.toString(result);
} catch (IllegalArgumentException e) {
return "错误:" + e.getMessage();
}
}
private double evaluate(String expression) {
Stack<Double> operands = new Stack<>();
Stack<Character> operators = new Stack<>();
int i = 0;
while (i < expression.length()) {
char c = expression.charAt(i);
if (Character.isDigit(c) || c == '.') {
StringBuilder operandBuilder = new StringBuilder();
while (i < expression.length() && (Character.isDigit(expression.charAt(i)) || expression.charAt(i) == '.')) {
operandBuilder.append(expression.charAt(i));
i++;
}
double operand = Double.parseDouble(operandBuilder.toString());
operands.push(operand);
} else if (c == '+' || c == '-' || c == '*' || c == '/' || c == '%') {
while (!operators.isEmpty() && hasHigherOrEqualPrecedence(c, operators.peek())) {
evaluateTop(operands, operators);
}
operators.push(c);
i++;
} else if (c == '(') {
operators.push(c);
i++;
} else if (c == ')') {
while (!operators.isEmpty() && operators.peek() != '(') {
evaluateTop(operands, operators);
}
if (operators.isEmpty()) {
throw new IllegalArgumentException("表达式包含未匹配的右括号");
}
operators.pop();
i++;
} else {
throw new IllegalArgumentException("表达式包含无效字符 " + c);
}
}
while (!operators.isEmpty()) {
evaluateTop(operands, operators);
}
if (operands.size() != 1 || !operators.isEmpty()) {
throw new IllegalArgumentException("表达式无效");
}
return operands.pop();
}
private boolean hasHigherOrEqualPrecedence(char operator1, char operator2) {
return (operator1 == '*' || operator1 == '/' || operator1 == '%') || (operator1 == '+' || operator1 == '-') && (operator2 == '+' || operator2 == '-');
}
private void evaluateTop(Stack<Double> operands, Stack<Character> operators) {
double operand2 = operands.pop();
double operand1 = operands.pop();
char operator = operators.pop();
double result = applyOperator(operator, operand1, operand2);
operands.push(result);
}
private double applyOperator(char operator, double operand1, double operand2) {
switch (operator) {
case '+':
return operand1 + operand2;
case '-':
return operand1 - operand2;
case '*':
return operand1 * operand2;
case '/':
if (operand2 == 0) {
throw new IllegalArgumentException("除数不能为零");
}
return operand1 / operand2;
case '%':
if (operand2 == 0) {
throw new IllegalArgumentException("除数不能为零");
}
return operand1 % operand2;
default:
throw new IllegalArgumentException("无效操作符 " + operator);
}
}
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
if (command.equals("=")) {
String expression = displayTextField.getText();
String result = calculate(expression);
displayTextField.setText(result);
} else {
displayTextField.setText(displayTextField.getText() + command);
}
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
calculator.setSize(300, 400);
calculator.setLocationRelativeTo(null);
calculator.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
calculator.setVisible(true);
}
}
```
该代码中使用了图形用户界面,包含数字、操作符和等号按钮,以及一个文本框来显示表达式和结果。当用户点击等号按钮时,程序会调用 `calculate` 方法来计算表达式的值,然后将结果显示在文本框中。 `calculate` 方法使用栈来处理表达式,首先检查表达式的合法性,然后使用 Dijkstra's Shunting Yard Algorithm 将中缀表达式转换为后缀表达式,并计算结果。
阅读全文
相关推荐
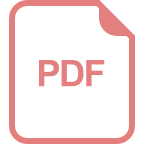
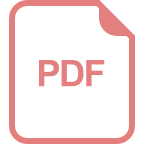
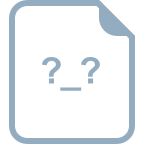
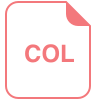
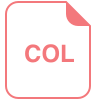



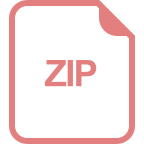
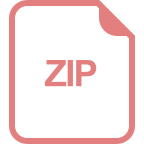