Vue3静态页面数据点击每一行数据跳转页面展示不同数据
时间: 2023-08-05 08:08:49 浏览: 49
可以通过 Vue Router 实现页面跳转和展示不同数据的功能。具体步骤如下:
1. 安装 Vue Router
在终端中运行以下命令:
```
npm install vue-router
```
2. 创建路由
在项目中创建一个 `router.js` 文件,定义路由:
```javascript
import { createRouter, createWebHistory } from 'vue-router'
import Home from './views/Home.vue'
import Detail from './views/Detail.vue'
const routes = [
{
path: '/',
name: 'Home',
component: Home
},
{
path: '/detail/:id',
name: 'Detail',
component: Detail,
props: true
}
]
const router = createRouter({
history: createWebHistory(),
routes
})
export default router
```
这里定义了两个路由,一个是首页 `/`,一个是详情页 `/detail/:id`,其中 `:id` 表示参数,用于动态展示不同的数据。
3. 创建组件
在项目中创建 `Home.vue` 和 `Detail.vue` 两个组件,分别用于展示首页和详情页的内容。
4. 在首页中展示数据
在 `Home.vue` 中展示数据,并将每一行数据包装在 `router-link` 中,用于跳转到详情页:
```html
<template>
<div>
<h1>首页</h1>
<ul>
<li v-for="item in list" :key="item.id">
<router-link :to="'/detail/' + item.id">{{ item.title }}</router-link>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
list: [
{ id: 1, title: '文章1', content: '这是文章1的内容' },
{ id: 2, title: '文章2', content: '这是文章2的内容' },
{ id: 3, title: '文章3', content: '这是文章3的内容' }
]
}
}
}
</script>
```
5. 在详情页中展示数据
在 `Detail.vue` 中展示传递过来的参数对应的数据:
```html
<template>
<div>
<h1>详情页</h1>
<p>{{ content }}</p>
</div>
</template>
<script>
export default {
props: {
id: {
type: Number,
required: true
}
},
data() {
return {
content: ''
}
},
created() {
// 根据传递过来的 id 获取对应的数据
this.content = this.getContentById(this.id)
},
methods: {
getContentById(id) {
// 根据 id 获取对应的数据,这里只是模拟数据
const data = {
1: { id: 1, title: '文章1', content: '这是文章1的内容' },
2: { id: 2, title: '文章2', content: '这是文章2的内容' },
3: { id: 3, title: '文章3', content: '这是文章3的内容' }
}
return data[id].content
}
}
}
</script>
```
6. 在入口文件中使用路由
在入口文件 `main.js` 中使用路由,并将其挂载到 Vue 实例中:
```javascript
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
createApp(App).use(router).mount('#app')
```
现在,点击首页中的每一行数据都可以跳转到对应的详情页,并展示不同的数据。
相关推荐
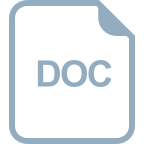














