用c语言创建一个顺序表,有输入,查询,取值,插入,删除,输出的功能
时间: 2024-05-30 14:16:17 浏览: 85
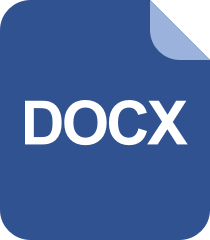
顺序表输入、查找、插入、删除

#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int length;
} SeqList;
void initList(SeqList *list) {
list->length = 0;
}
void insert(SeqList *list, int position, int value) {
if (list->length == MAX_SIZE) {
printf("List is full.\n");
return;
}
if (position < 1 || position > list->length + 1) {
printf("Invalid position.\n");
return;
}
int i;
for (i = list->length; i >= position; i--) {
list->data[i] = list->data[i - 1];
}
list->data[position - 1] = value;
list->length++;
}
void delete(SeqList *list, int position) {
if (position < 1 || position > list->length) {
printf("Invalid position.\n");
return;
}
int i;
for (i = position - 1; i < list->length - 1; i++) {
list->data[i] = list->data[i + 1];
}
list->length--;
}
void printList(SeqList list) {
int i;
for (i = 0; i < list.length; i++) {
printf("%d ", list.data[i]);
}
printf("\n");
}
int get(SeqList list, int position) {
if (position < 1 || position > list.length) {
printf("Invalid position.\n");
return -1;
}
return list.data[position - 1];
}
int search(SeqList list, int value) {
int i;
for (i = 0; i < list.length; i++) {
if (list.data[i] == value) {
return i + 1;
}
}
return -1;
}
int main() {
SeqList list;
initList(&list);
int choice, position, value;
while (1) {
printf("1. Insert\n2. Delete\n3. Print\n4. Get\n5. Search\n6. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter the position and value: ");
scanf("%d%d", &position, &value);
insert(&list, position, value);
break;
case 2:
printf("Enter the position: ");
scanf("%d", &position);
delete(&list, position);
break;
case 3:
printList(list);
break;
case 4:
printf("Enter the position: ");
scanf("%d", &position);
printf("Value: %d\n", get(list, position));
break;
case 5:
printf("Enter the value: ");
scanf("%d", &value);
printf("Position: %d\n", search(list, value));
break;
case 6:
exit(0);
default:
printf("Invalid choice.\n");
}
}
return 0;
}
阅读全文
相关推荐















