穿越沙漠问题matlab代码
时间: 2023-09-10 07:13:43 浏览: 147
以下是使用Matlab实现Dijkstra算法解决穿越沙漠问题的示例代码:
```matlab
function shortest_distance = dijkstra(graph, start, end)
% 初始化距离矩阵和堆
distances = Inf(length(graph), 1);
distances(start) = 0;
heap = [0, start];
% 开始搜索
while ~isempty(heap)
% 从堆中取出距离最小的节点
[current_distance, current_vertex] = heappop(heap);
% 如果当前节点已经被访问过,则跳过
if current_distance > distances(current_vertex)
continue;
end
% 遍历当前节点的相邻节点
neighbors = find(graph(current_vertex, :) > 0);
for i = 1:length(neighbors)
neighbor = neighbors(i);
weight = graph(current_vertex, neighbor);
% 计算从起点到相邻节点的距离
distance = current_distance + weight;
% 如果距离比已知的距离更短,则更新距离
if distance < distances(neighbor)
distances(neighbor) = distance;
% 将相邻节点加入堆中
heap = heapinsert(heap, [distance, neighbor]);
end
end
end
% 返回起点到终点的最短距离
shortest_distance = distances(end);
end
% 示例数据
graph = [0, 5, 2, 0; 0, 0, 0, 4; 0, 8, 0, 7; 0, 0, 0, 0];
start = 1;
end = 4;
% 使用Dijkstra算法求解起点到终点的最短距离
shortest_distance = dijkstra(graph, start, end);
disp(['最短距离为:', num2str(shortest_distance)]);
```
在上述代码中,我们使用矩阵来存储图的邻接矩阵,其中每个元素表示相邻节点之间的权值。然后,我们使用堆(heap)来存储待访问的节点,每次从堆中取出距离最小的节点进行访问,同时更新与该节点相邻的节点的距离。最后,我们返回起点到终点的最短距离。需要注意的是,Matlab中的堆操作需要使用`heapq`库中的函数进行实现,因此需要在代码中调用相关函数。
阅读全文
相关推荐



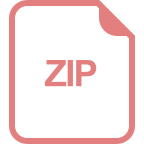
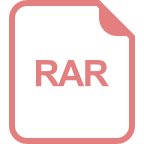
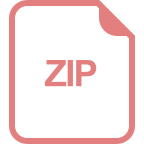
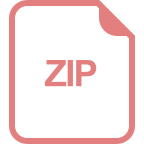
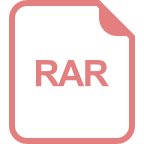
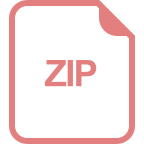
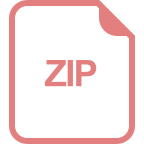
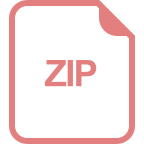
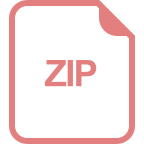
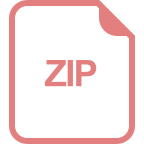
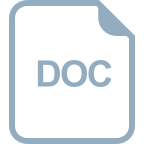