python爬取百度贴吧中原工学院吧首页的标题
时间: 2023-06-27 20:06:14 浏览: 212
可以使用Python中的requests和BeautifulSoup库来爬取百度贴吧中某个贴吧的标题。
以下是一个示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 获取原工学院贴吧首页的HTML内容
url = 'http://tieba.baidu.com/f?kw=%E5%8C%97%E4%BA%AC%E7%90%86%E5%B7%A5%E5%A4%A7%E5%AD%A6&ie=utf-8&pn=0'
response = requests.get(url)
html = response.content
# 解析HTML内容,获取帖子标题
soup = BeautifulSoup(html, 'html.parser')
titles = soup.select('a.j_th_tit')
# 输出帖子标题
for title in titles:
print(title.get_text())
```
注意,这里的`select()`方法是使用CSS选择器来选择元素的方法,`'a.j_th_tit'`表示选择class为`j_th_tit`的`a`标签元素,即帖子标题。
阅读全文
相关推荐
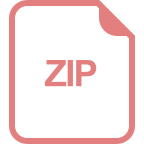
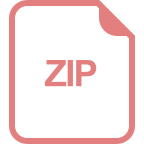
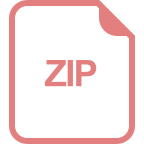
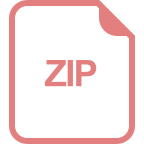
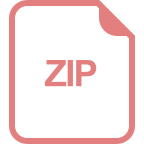
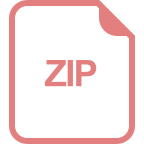
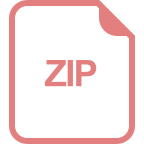
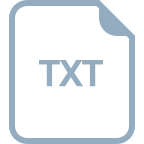
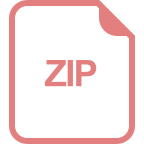
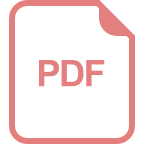
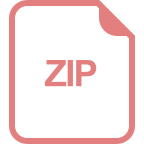
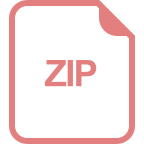
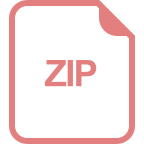