python 如何实现缓存
时间: 2023-10-02 22:11:31 浏览: 54
Python 可以使用以下方式实现缓存:
1. 使用 Python 内置的 `lru_cache` 装饰器,将函数的执行结果缓存起来,以减少函数的重复计算。例如:
```python
from functools import lru_cache
@lru_cache(maxsize=None)
def my_func(arg):
# some computation
return result
```
2. 使用 Python 的 `dict` 类型作为缓存容器,以键值对的方式存储函数的输入参数和输出结果。例如:
```python
cache = {}
def my_func(arg):
if arg in cache:
return cache[arg]
else:
result = # some computation
cache[arg] = result
return result
```
3. 使用 Python 的第三方缓存库,例如 `redis`、`memcached` 等,将函数的输入参数作为缓存键,输出结果作为缓存值,以加速函数的执行。例如:
```python
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
def my_func(arg):
cache_key = f'my_cache_{arg}'
if r.exists(cache_key):
return r.get(cache_key)
else:
result = # some computation
r.set(cache_key, result)
return result
```
相关推荐
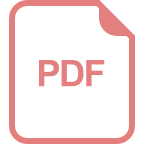
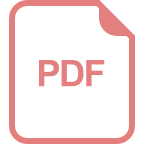
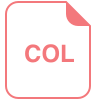
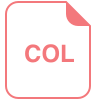
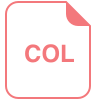
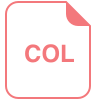
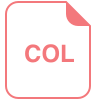









